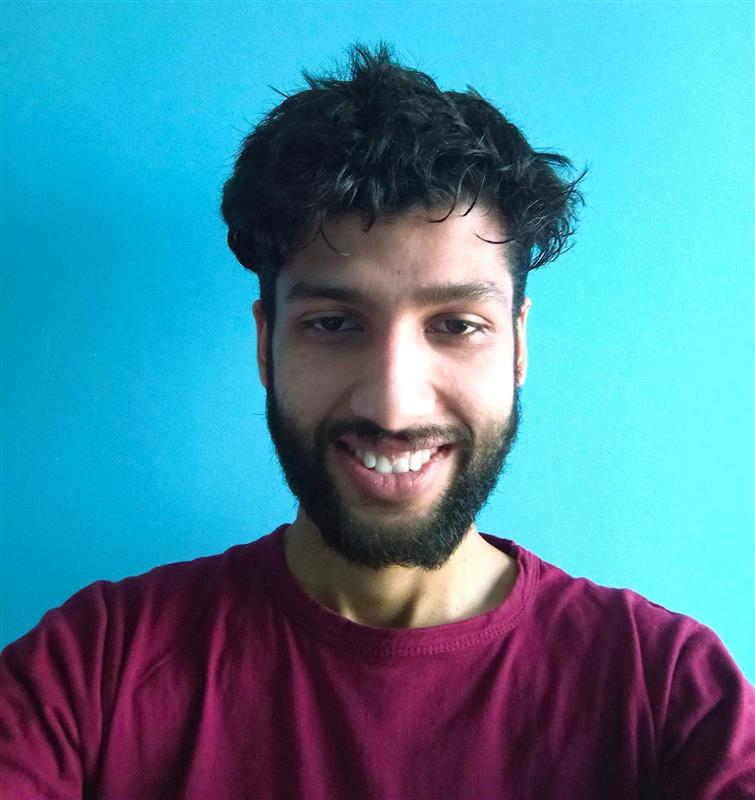
Abhilekh Gautam
System Engineer
Answered 7 questions
About
Hi, I am Abhilekh. I currently work as a System Engineer. My primary interest is in Compilers and Programming language implementation. I like to understand how computer programs work under the hood. I enjoy reading books, solving problems, analyzing algorithms, and staying up to date with modern advancements in computing technologies.
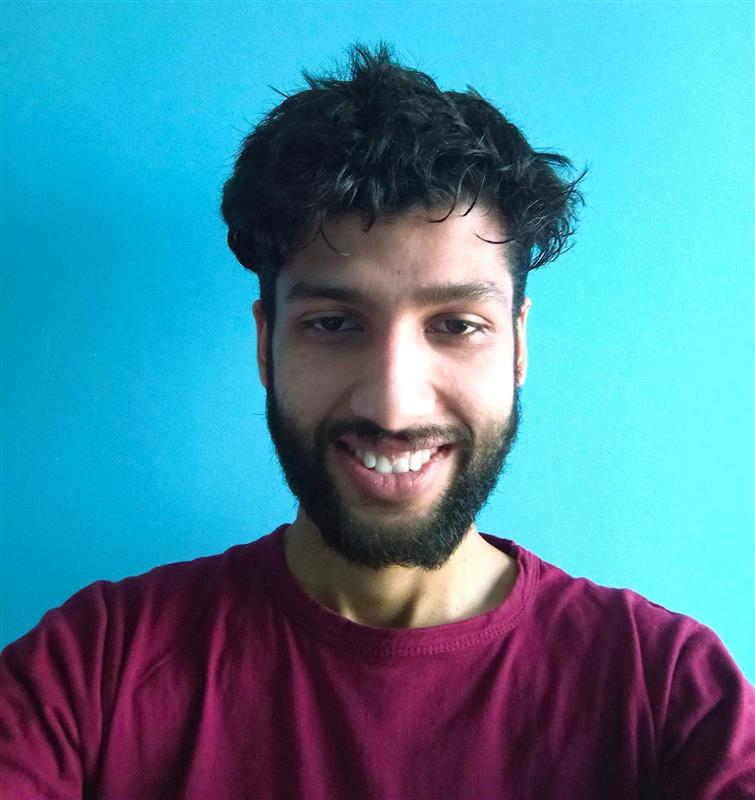
printf()
is used to display text or values on the screen.
For Example:
printf("Hello, World");
The above code displays the text Hello, World
on the screen.
I hope this clears your confusion. If you have more questions feel free to ask!
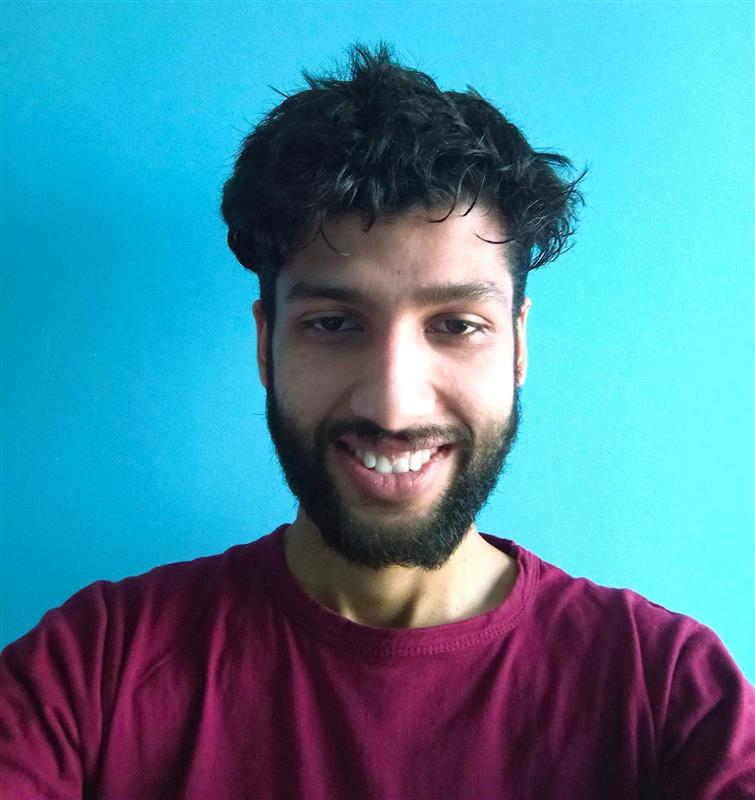
In C, scanf()
is used to read input from the user, while printf()
is used to display output on the screen.
Think of it this way:
scanf()
receives data (input)printf()
sends data to the screen (output)
Example:
#include
int main() {
int age;
printf("Enter your age: "); // Output
scanf("%d", &age); // Input
printf("You are %d years old.", age); // Output
return 0;
}
Here, printf()
first prompts the user, and then scanf()
captures the input into the age
variable. After that, printf()
is used again to show the result.
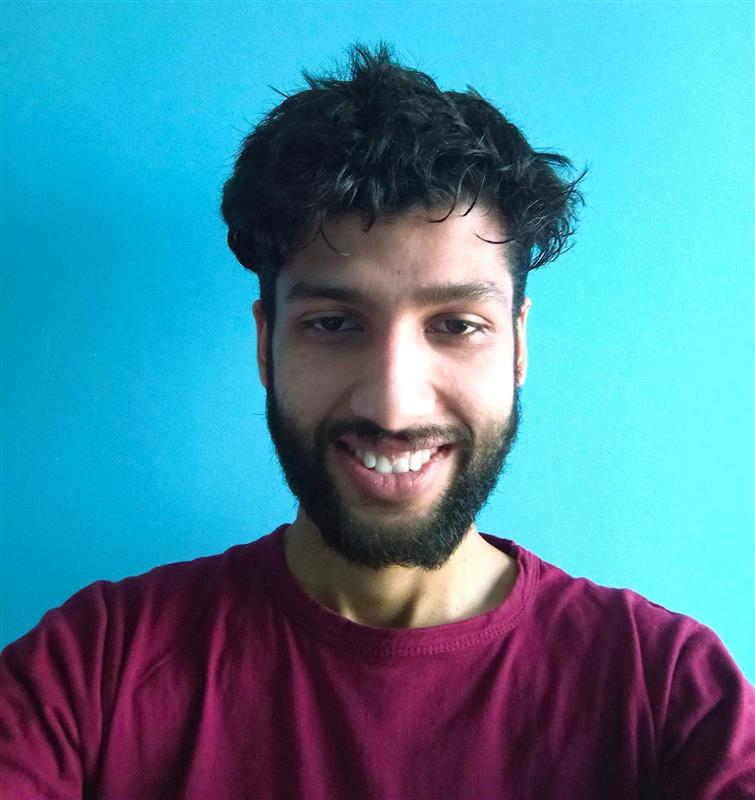
Java code can run on any operating system without needing changes because of a key component called the Java Virtual Machine (JVM).
Think of the JVM as a translator or a middle layer. Here's how it works:
When you write Java code, it’s compiled into an intermediate form called bytecode, not directly into machine code for a specific OS.
This bytecode is platform-independent—meaning it's the same no matter where it's run.
The JVM on each device reads the bytecode and translates it into machine code that the specific operating system and hardware can understand.
Since each OS (Windows, macOS, Linux, etc.) has its own version of the JVM, your Java program doesn't need to change. The JVM handles all the OS-specific translation for you.
Example:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Once compiled, this code becomes bytecode in a .class
file. That same file can be run on any system that has a JVM installed—no changes required.
This is what people mean when they say "Write once, run anywhere", which is one of Java’s biggest strengths.
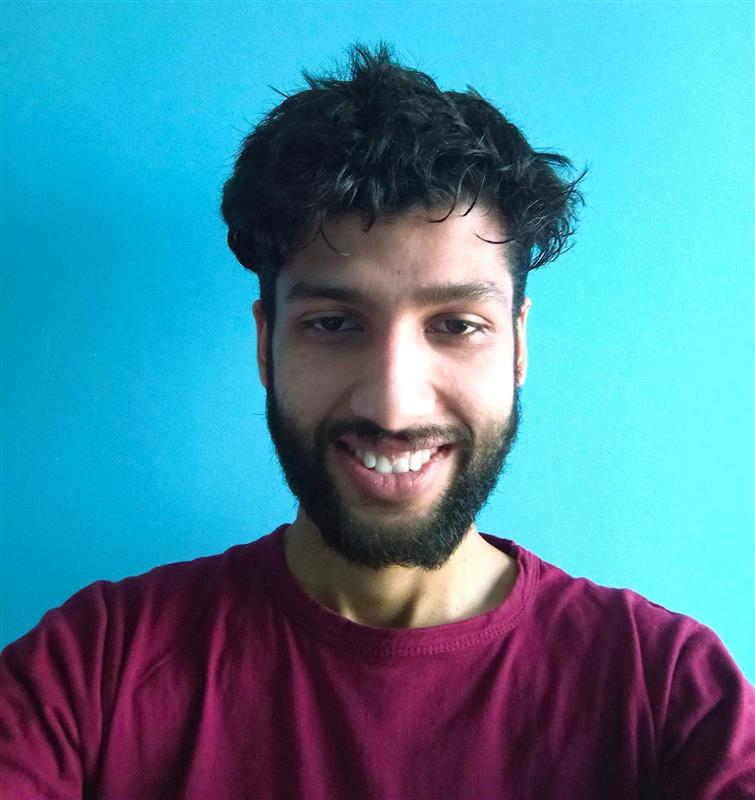
Python and C are different programming languages. Python is easier to learn and use, especially for beginners, because it reads almost like plain English. C is a bit harder because it requires more attention to details.
Comparison between Python and C
Python requires only one easy-to-read line to print something:
print("Hello, World!")
C requires you to manage more setup, like including libraries (#include
) and defining a main
function:
#include
int main() {
printf("Hello, World!\n");
return 0;
}
Key Differences:
Syntax Simplicity: Python is simpler and shorter; C needs more boilerplate code.
Speed: C programs generally run faster because they are closer to "machine language."
Use Cases: Python is often used for web development, automation, AI, and data science. C is used where performance matters a lot, like in operating systems, embedded systems, and hardware drivers.
Memory Management: In C, you must manage memory manually. In Python, memory management is automatic (through garbage collection).
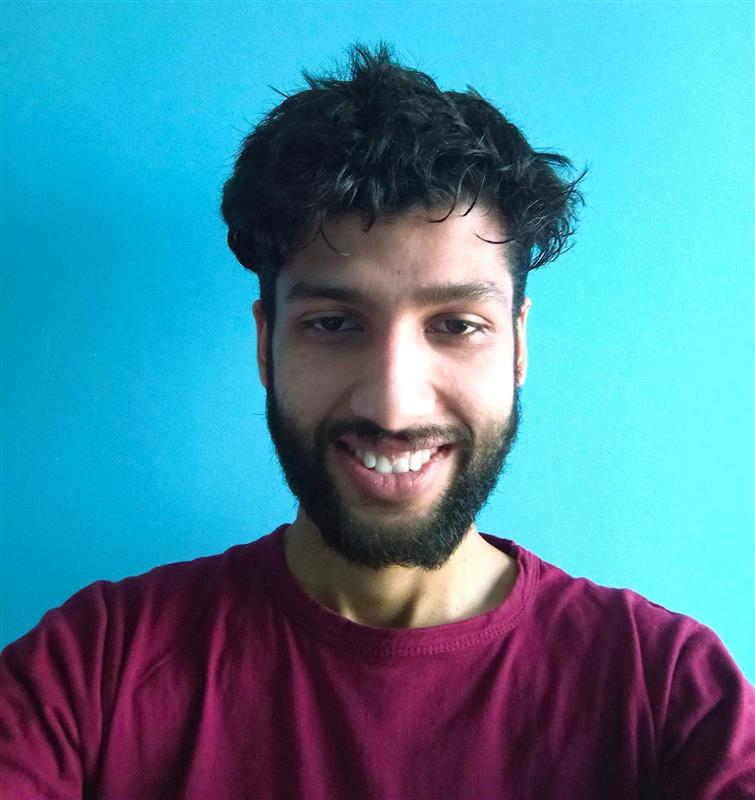
For integers, the format specifier is %d
. For other types:
- float:
%f
- double:
%lf
- char:
%c
You’ll learn more about these and how to use them later in the course, so don’t worry about it for now.
Keep going, you’re doing great!
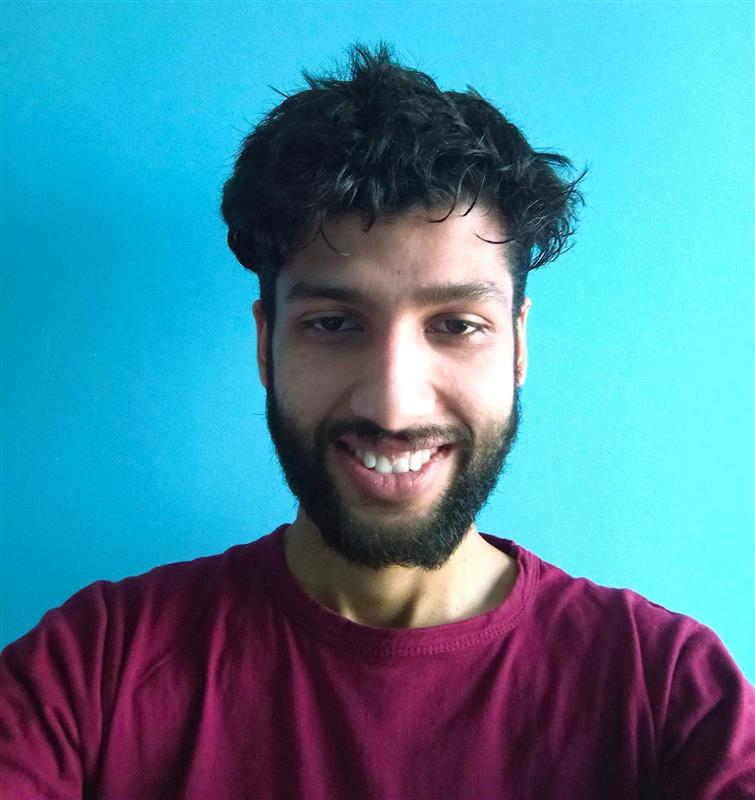
Generally, there are two types of loops in Python:
- for Loop
- while Loop
If you want to learn more about loops, I suggest you check out the Decision Making & Loops chapter.
Also note that, to offer you best help, we focus on answering questions specific to the page or course. So if you have such questions, feel free to ask. I'm happy to help.
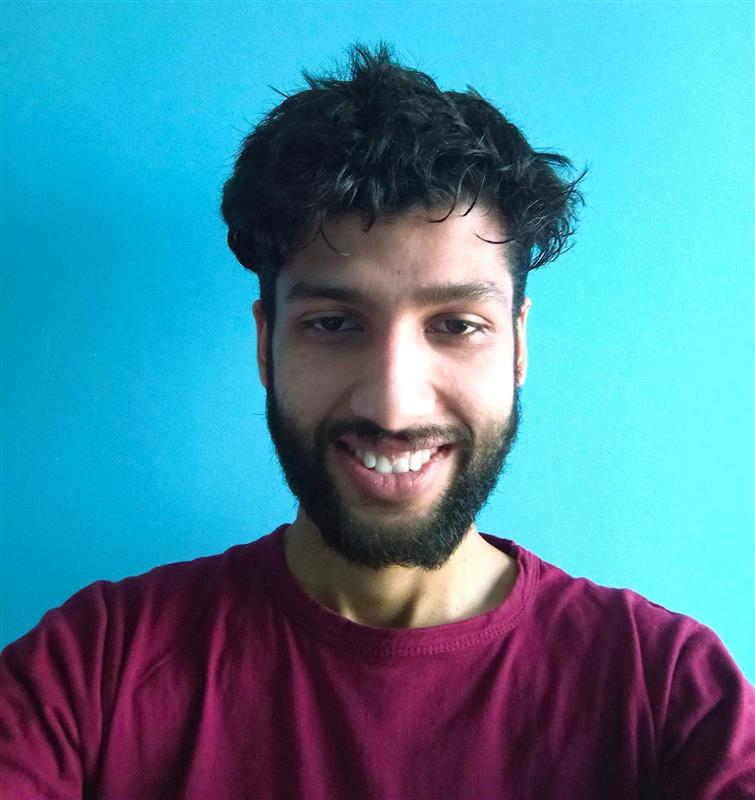
Yes, C and C++ are different, though closely related. C++ is essentially an extension of the C programming language—it builds on C’s features and adds additional tools, especially object-oriented programming (OOP).
Here’s a quick breakdown:
C is a procedural programming language, meaning it focuses on functions and sequences of instructions.
C++ supports everything C does, but also includes object-oriented features like classes, inheritance, and polymorphism, which allow for more structured and reusable code.
Example in C (procedural approach):
#include
void greet() {
printf("Hello, world!");
}
int main() {
greet();
return 0;
}
Same idea in C++ (with an object-oriented approach):
#include
using namespace std;
class Greeter {
public:
void greet() {
cout << "Hello, world!";
}
};
int main() {
Greeter g;
g.greet();
return 0;
}
So while the basics (like variables, loops, and syntax) are very similar, C++ gives you more tools and structure—especially useful for large, complex programs.