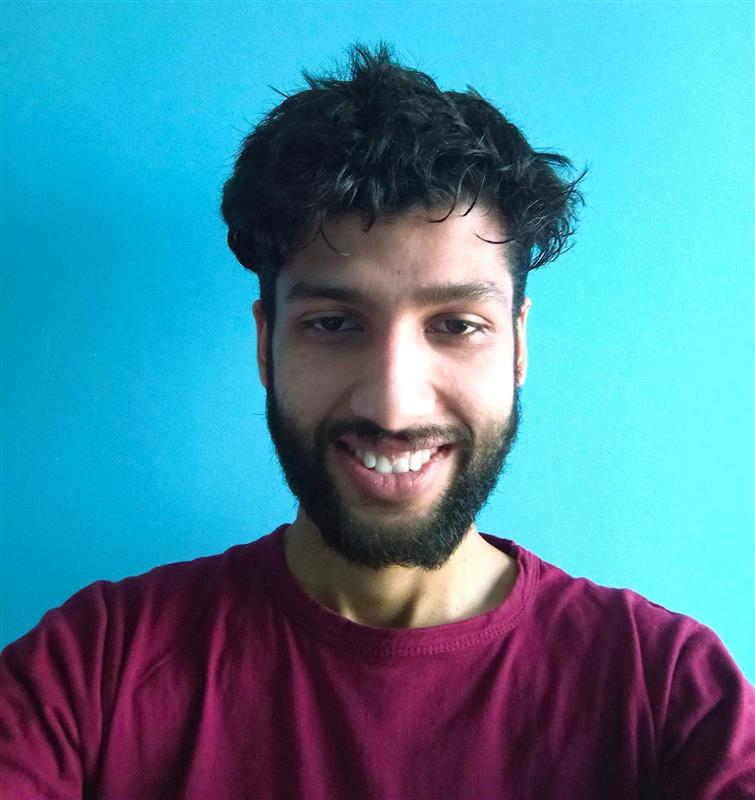
Yes, C and C++ are different, though closely related. C++ is essentially an extension of the C programming language—it builds on C’s features and adds additional tools, especially object-oriented programming (OOP).
Here’s a quick breakdown:
C is a procedural programming language, meaning it focuses on functions and sequences of instructions.
C++ supports everything C does, but also includes object-oriented features like classes, inheritance, and polymorphism, which allow for more structured and reusable code.
Example in C (procedural approach):
#include
void greet() {
printf("Hello, world!");
}
int main() {
greet();
return 0;
}
Same idea in C++ (with an object-oriented approach):
#include
using namespace std;
class Greeter {
public:
void greet() {
cout << "Hello, world!";
}
};
int main() {
Greeter g;
g.greet();
return 0;
}
So while the basics (like variables, loops, and syntax) are very similar, C++ gives you more tools and structure—especially useful for large, complex programs.