Ask Programiz - Human Support for Coding Beginners
Explore questions from fellow beginners answered by our human experts. Have some doubts about coding fundamentals? Enroll in our course and get personalized help right within your lessons.
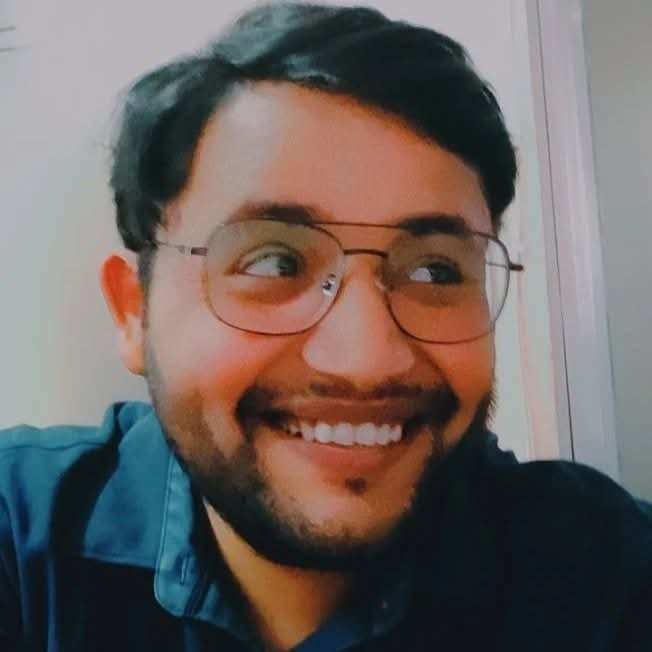
Hi Abhishek!
For starters, we believe that anyone can code; all you need is study and practice.
Transitioning from electrical engineering to the computer science sector can be a good move. Your electrical engineering background is actually a huge advantage; you already know how to break down complex problems.
As for whether coding is the right fit for you, consider this:
It looks like you've just started the Python Basics course. Continue through it, take the time to practice writing code, and see how you feel about it. If you enjoy solving problems and building projects, that's a good sign that coding could be a fulfilling path for you.
Let me know if you have any more questions or need guidance along the way. I'm here to help!
Oh, and one last thing - if you're willing to put in the time, if you're willing to study and practice, there's absolutely no reason why someone from your background can't excel in coding.
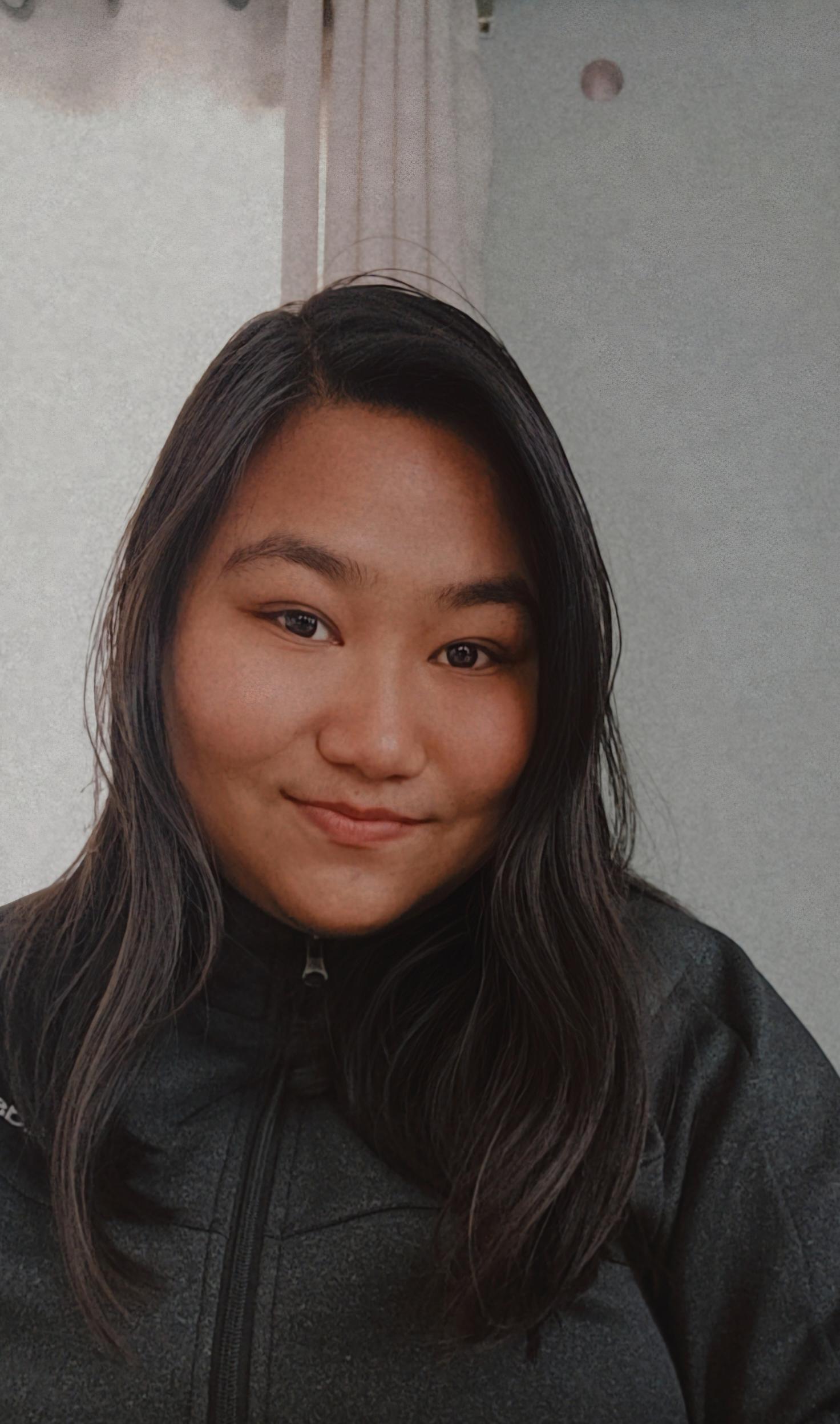
Hey Bisman! 😊
That’s such a thoughtful question — and you're not alone in wondering this!
HTML is called a markup language because its main job is to structure and display content on a webpage. It tells the browser things like:
- "This is a heading"
- "This is a paragraph"
- "This is a link or an image"
But HTML doesn't have logic — it can't make decisions, repeat actions, or respond to conditions like a programming language can.
For example, in a programming language (like Python or JavaScript), you can say:
if score > 50:
print("You passed!")
HTML can’t do that kind of logic — it just marks up content so the browser knows how to show it.
So in short: HTML structures content, but it doesn’t “think” or “decide” — that’s why it’s not considered a programming language. 😊
You're asking awesome questions — keep going! You're learning fast! 🚀💡
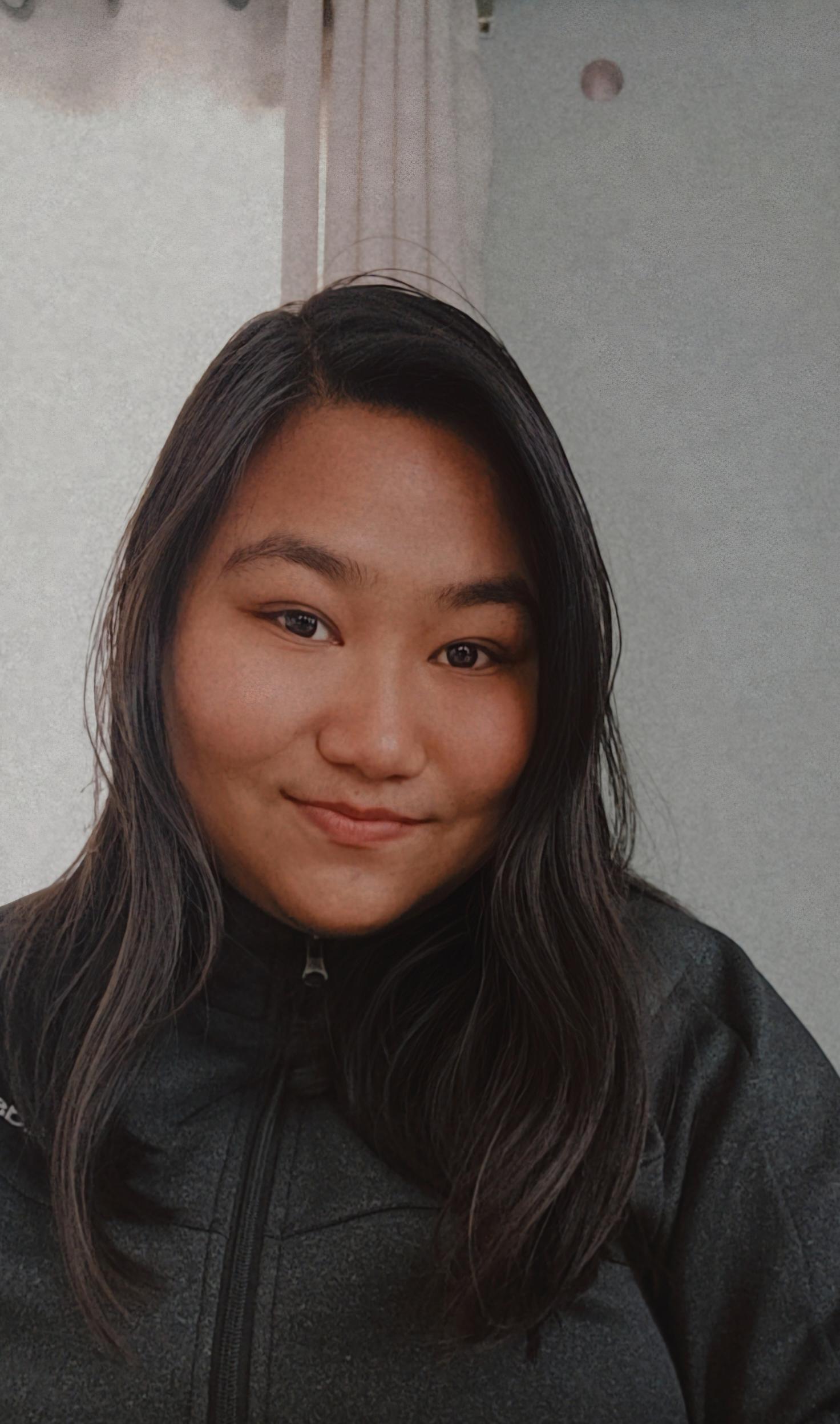
Hey! In programming, fixed values are values you write directly into your code — they don't change while the program runs. These are called literals.
For example:
5
→ an integer literal3.14
→ a floating-point (double) literal"Hello"
→ a string literal
They're called "fixed" because you're using them directly in your code, without assigning them to variables (yet).
Let me know if you want to see how to use them in a C++ program!

So, in Python, you don't need to use curly braces {}
when printing a variable like this:
name = input("Enter your name: ")
print("Your name is", name)
That works because print()
can take multiple things, separated by commas. It just puts spaces between them automatically, so you don't have to do anything fancy.
Now, curly braces do show up when you're using something like an f-string. That looks like this:
print(f"Your name is {name}")
In that case, the {name}
is inside the string, and Python replaces it with the actual value of the name
variable — but only because the string starts with an f
.
So yeah, curly braces are just for special formatting stuff like f-strings. If you're just printing normally with commas, you don’t need them at all.
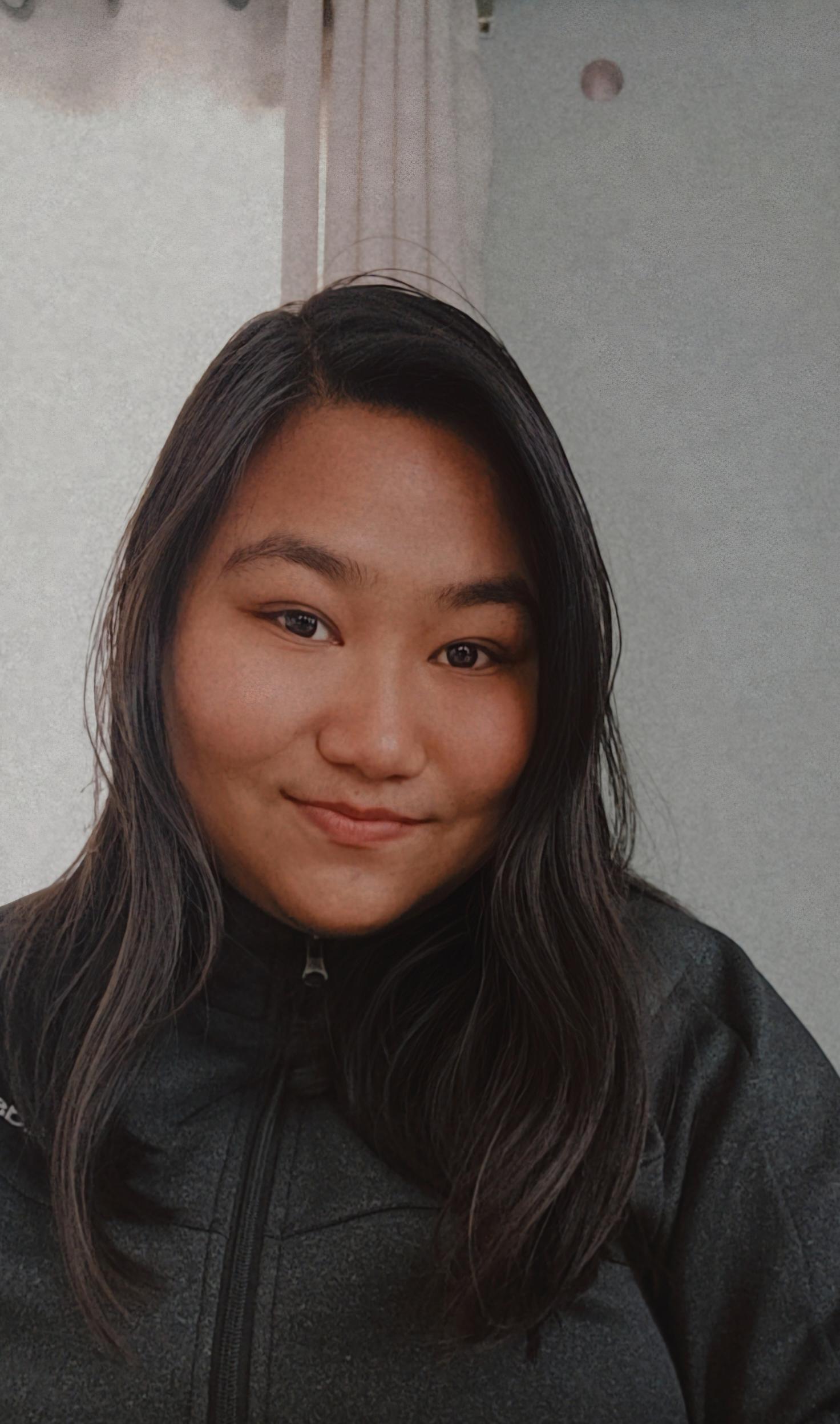
Whitespaces are just empty spaces in your text.
For example:
text = " Hello "
This has extra spaces before and after Hello
.
Those extra spaces are called whitespaces.
We usually remove them to make the text look clean.
So when you see something like , that little
/
just means the tag is self-closing — it doesn’t need an end tag like .
But guess what? Starting with HTML5, you don’t even need the /
anymore. You can just write:
And it works the same! Super simple. 👍
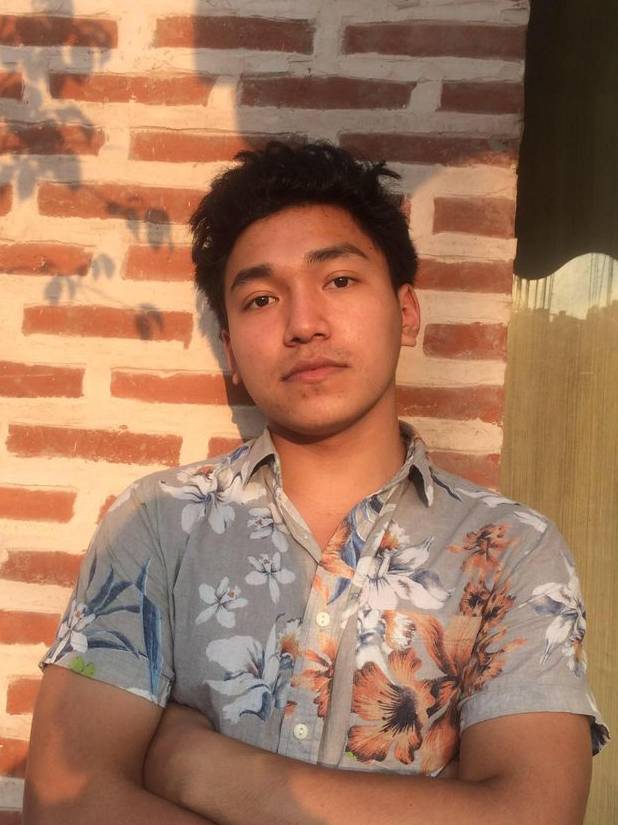
Simply put,
Memory location refers to a specific spot in the computer’s memory where data is stored. Think of it as a unique address where your data lives while your program is running. Every variable in your program is stored in one of these memory locations.
Data type is about the kind of data that goes into a memory location. For example, if you’re storing a number, the data type could be an int (integer) or a float (decimal number). If you’re storing text, the data type would be char or string.
So, in simple terms: Memory location is where data lives, and data type is what kind of data it is.
It’s okay if it's not 100% clear right now. As you continue with the course, this concept will make more sense.
Let me know if you need help with anything else.
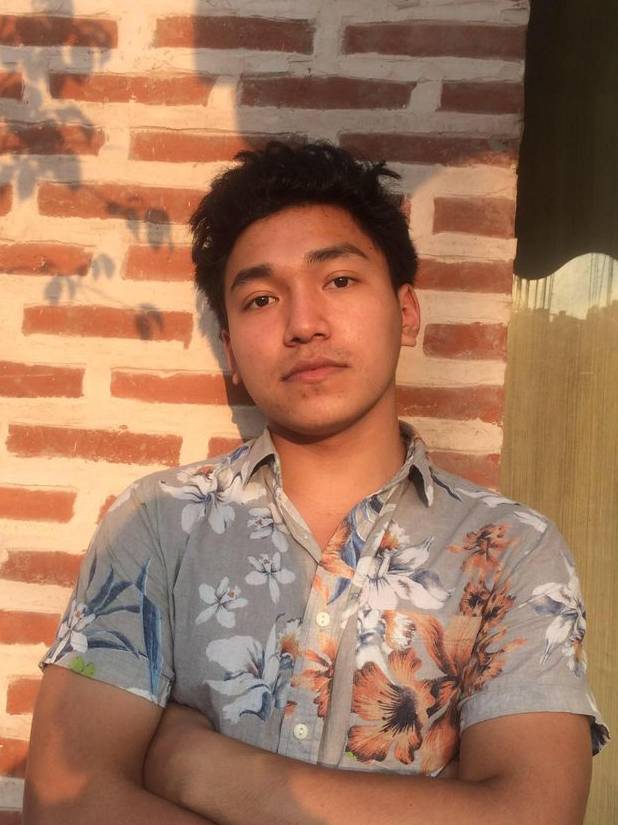
In programming, input simply means getting information from the user.
As you progress through the course, you'll learn how to take input in Python and use it in your programs.
Let me know if anything is unclear or if you'd like to explore this further.
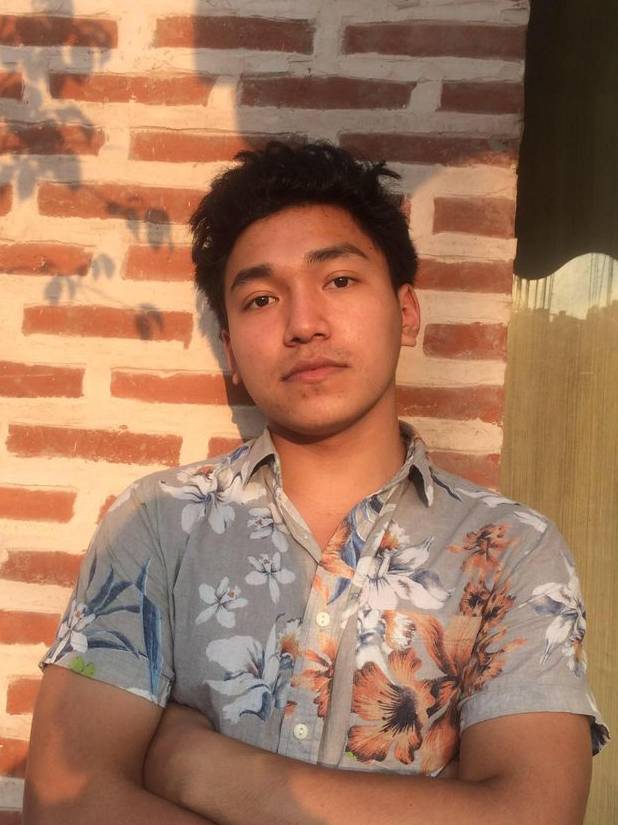
Yes, console.log()
in JavaScript is similar to print()
in Python — both are used to display information to the console or terminal.

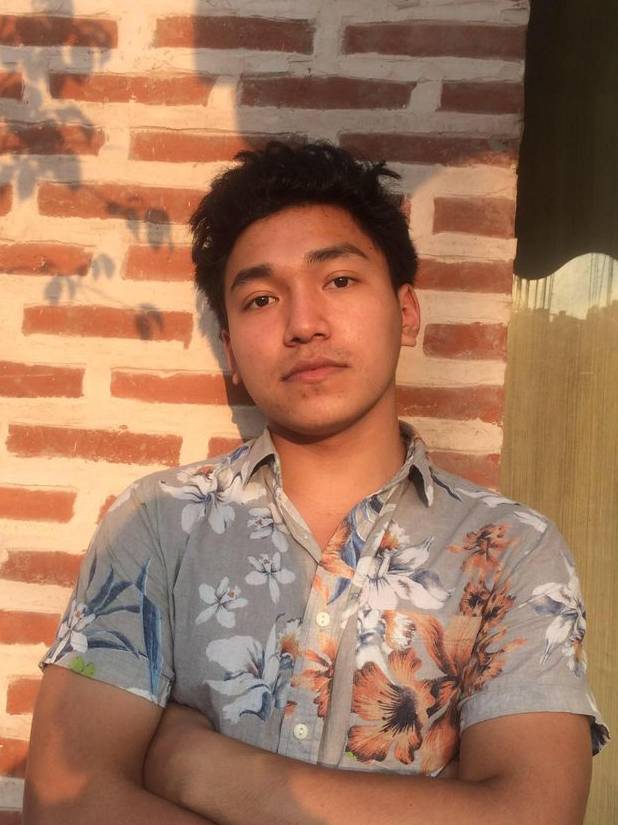
Since you already know how range()
works, let’s look at an example to understand how the step argument works.
Consider this code:
print(list(range(1, 6)))
Output
[1, 2, 3, 4, 5]
Here, the list goes up by 1
each time by default.
Now let’s add 2
as the step:
print(list(range(1, 6, 2)))
Output
[1, 3, 5]
In this case, the list still starts at 1
, but it jumps by 2
instead of 1
.
So, the step argument simply controls how much the value increases by in each step.
Let me know if you need more clarification on this.