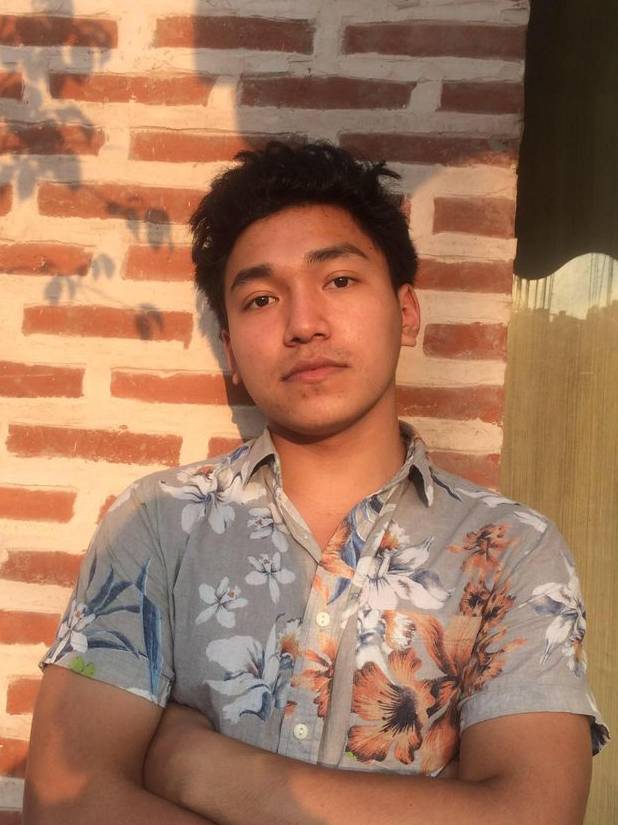
Kelish Rai
Technical Content Writer @Programiz
Answered 84 questions
About
Hi, I'm Kelish, Technical Content Writer at Programiz. I break down complex programming concepts and turn them into easy-to-understand articles, tutorials, and courses. I'm also a developer at heart—I love solving coding problems, exploring algorithms, and staying updated with the latest tech stuff. If I'm not writing content, there's a good chance I'm working on a side project.
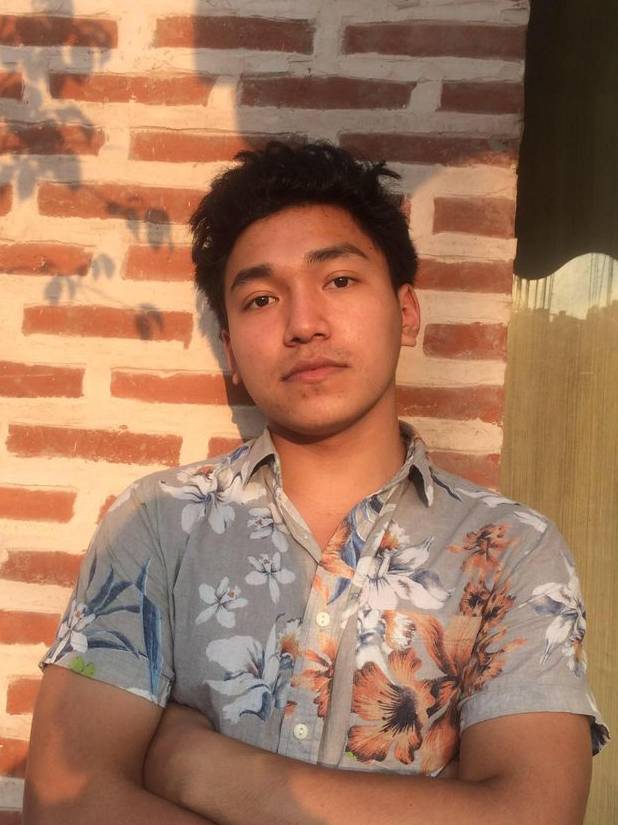
Yes, you can use Python to build apps, but how commonly it’s used depends on the type of app.
Python is really popular for web apps. A lot of websites and web services use Python behind the scenes, especially with frameworks like Django and Flask. So if you're interested in building web-based projects, Python is a great choice.
For desktop apps or mobile apps, Python is less commonly used. There are tools like Tkinter (for desktop apps) or Kivy and BeeWare (for mobile apps), but most developers usually prefer other languages like Swift for iOS, Kotlin for Android, or JavaScript frameworks for cross-platform apps because they offer more native features and smoother performance.
That said, Python is still great for learning app development concepts, automating tasks, building quick tools, and working on web projects.
If you’d like help getting started or if anything from the course needs clarification, just let me know. I’m happy to help.
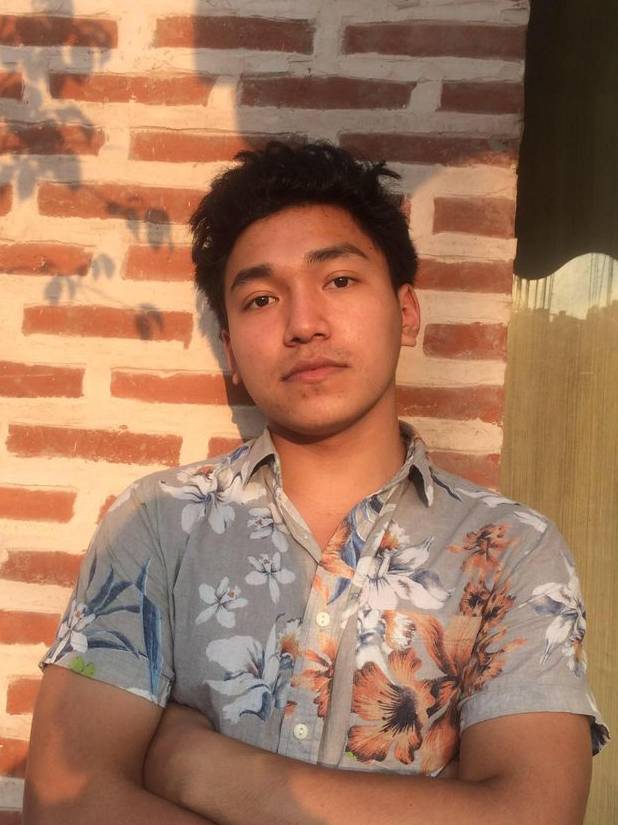
Simply put,
Memory location refers to a specific spot in the computer’s memory where data is stored. Think of it as a unique address where your data lives while your program is running. Every variable in your program is stored in one of these memory locations.
Data type is about the kind of data that goes into a memory location. For example, if you’re storing a number, the data type could be an int (integer) or a float (decimal number). If you’re storing text, the data type would be char or string.
So, in simple terms: Memory location is where data lives, and data type is what kind of data it is.
It’s okay if it's not 100% clear right now. As you continue with the course, this concept will make more sense.
Let me know if you need help with anything else.
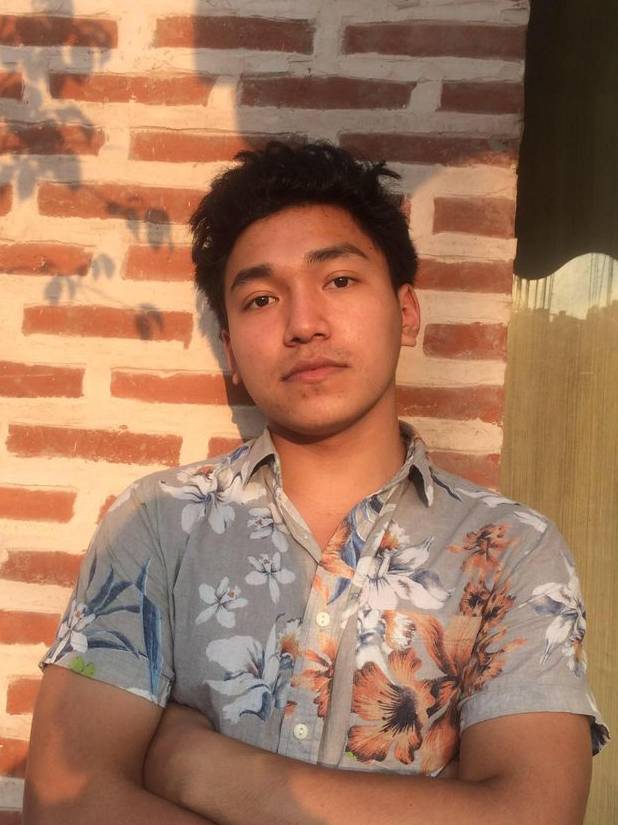
In programming, input simply means getting information from the user.
As you progress through the course, you'll learn how to take input in Python and use it in your programs.
Let me know if anything is unclear or if you'd like to explore this further.
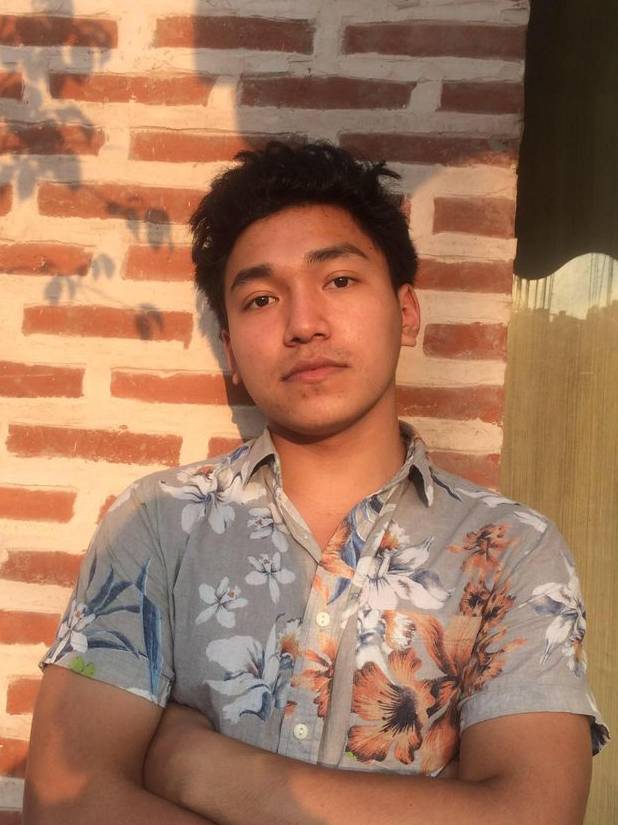
Yes, console.log()
in JavaScript is similar to print()
in Python — both are used to display information to the console or terminal.
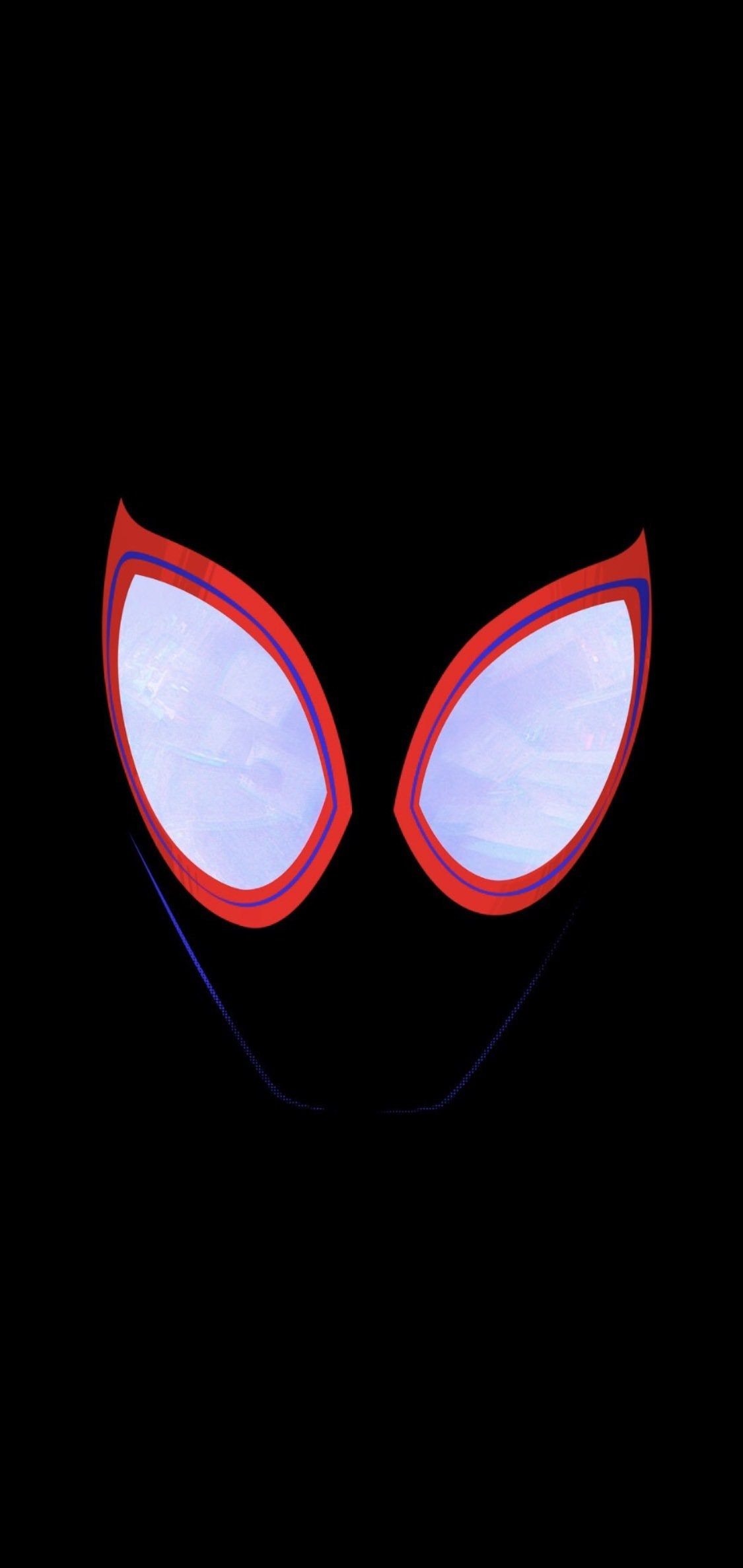
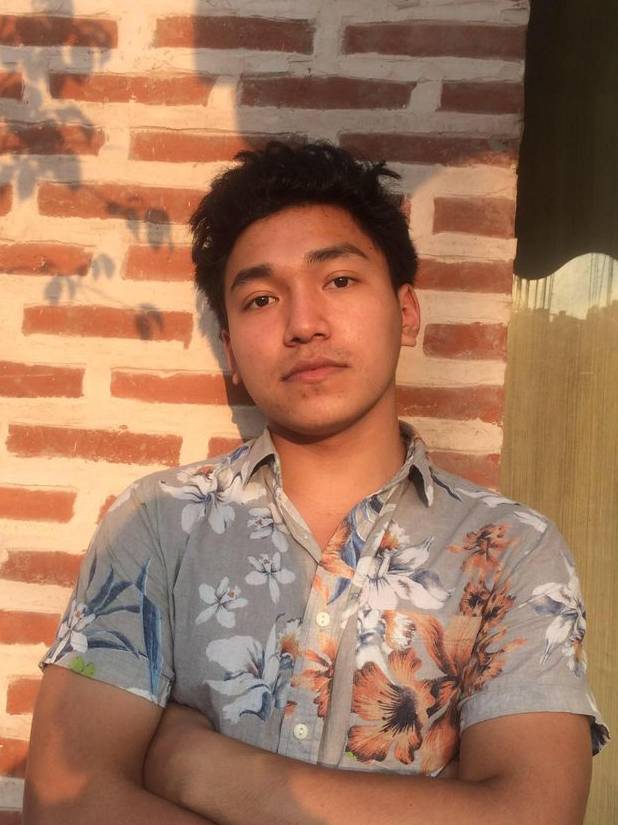
Since you already know how range()
works, let’s look at an example to understand how the step argument works.
Consider this code:
print(list(range(1, 6)))
Output
[1, 2, 3, 4, 5]
Here, the list goes up by 1
each time by default.
Now let’s add 2
as the step:
print(list(range(1, 6, 2)))
Output
[1, 3, 5]
In this case, the list still starts at 1
, but it jumps by 2
instead of 1
.
So, the step argument simply controls how much the value increases by in each step.
Let me know if you need more clarification on this.
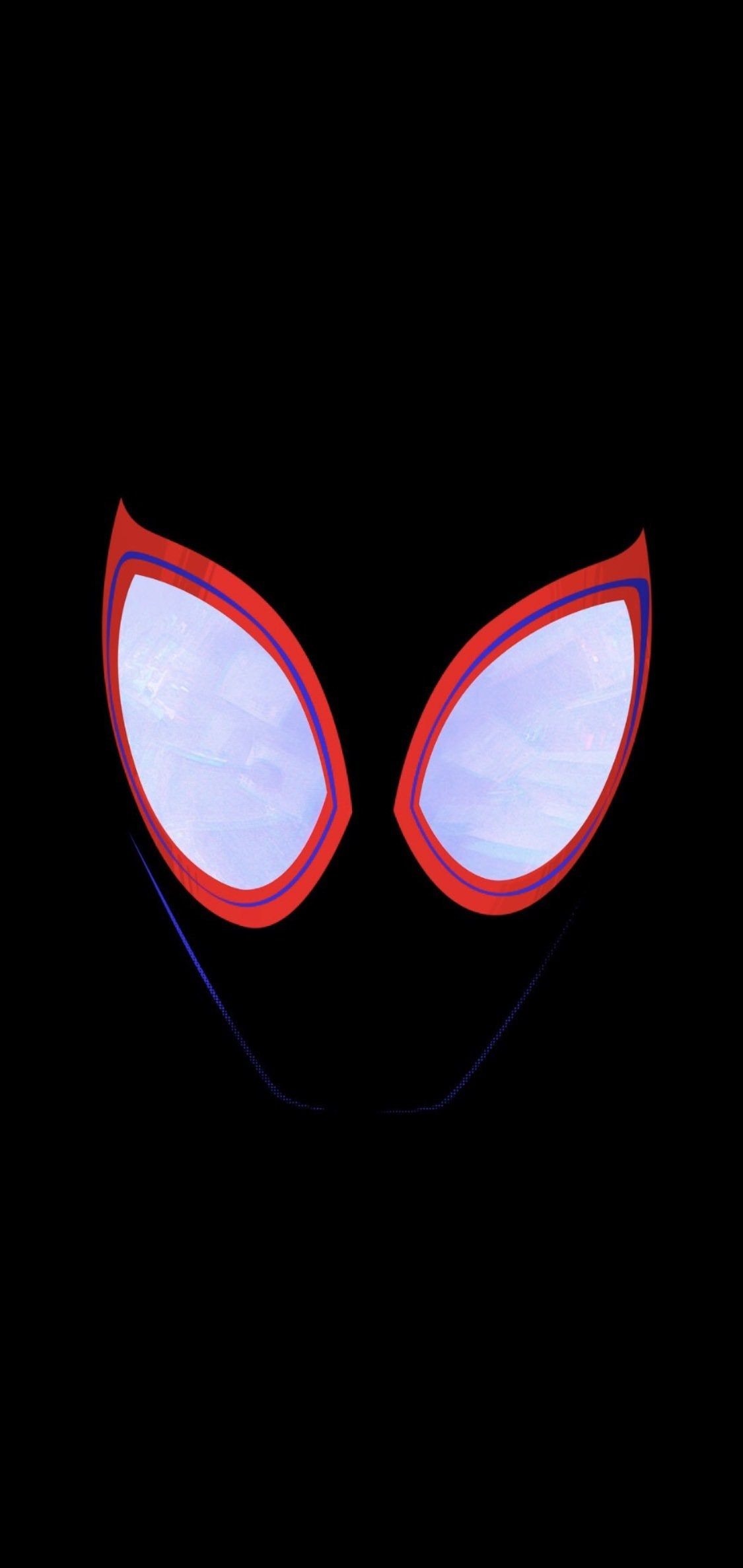
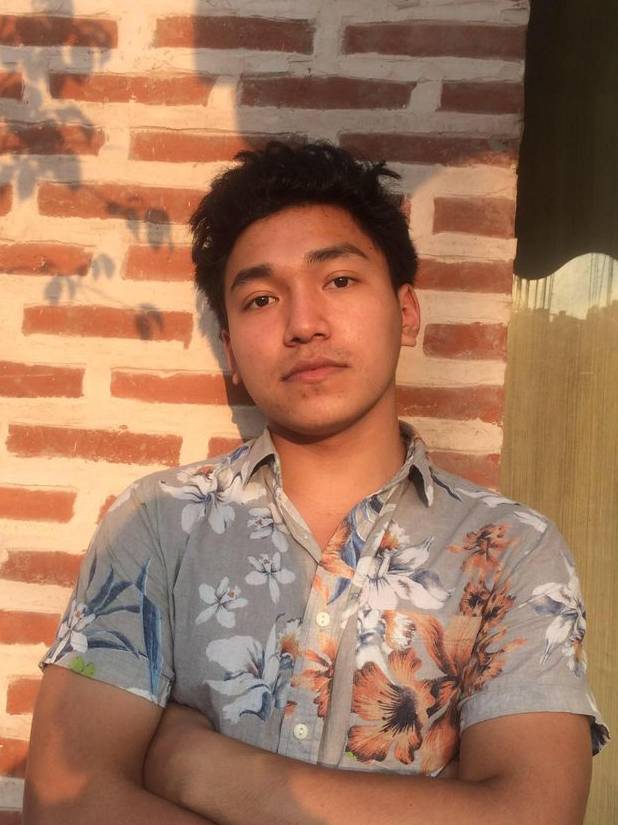
When you do range(1, 7)
, it gives you numbers starting from 1
up to but not including 7
. So it stops at 6
.
It’s not about indexing exactly — that’s just how range()
works in Python. The second number is treated as the "stop point", and it’s excluded from the result.
Let me know if you need more explanation on this. I'm happy to help.
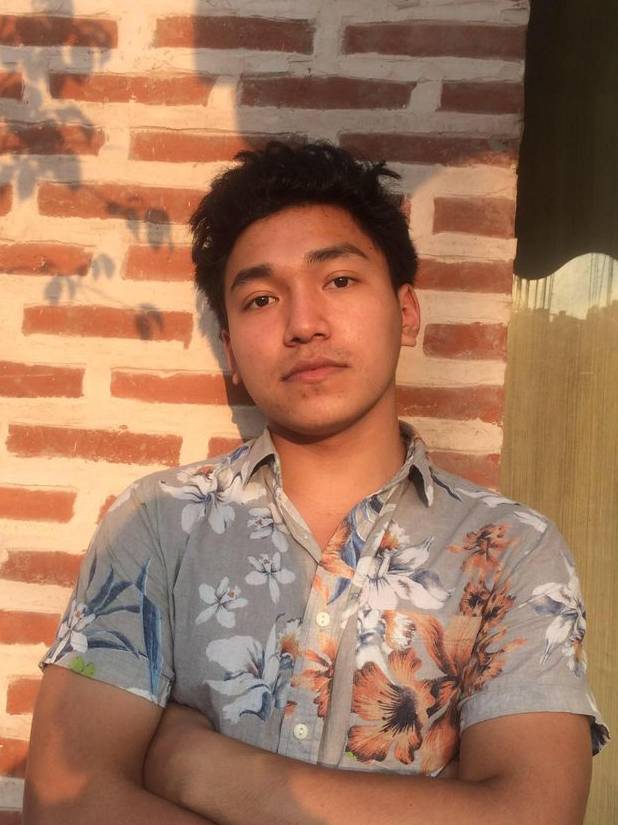
When performing arithmetic operations in Python, the result will be a floating-point number if you use the /
operator for division. For example, 5 / 2
will give you 2.5
.
If you're using operations like addition (+
), subtraction (-
), or multiplication (*
), the result will be in floating-point only if any of the values involved are floating-point numbers. For example, 5 + 2.2
will give you 7.2
.
If you use the //
operator for integer division, like 5 // 2
, the result will be an integer (in this case, 2
).
This distinction helps you get the result you expect, depending on the type of operation and the numbers involved.
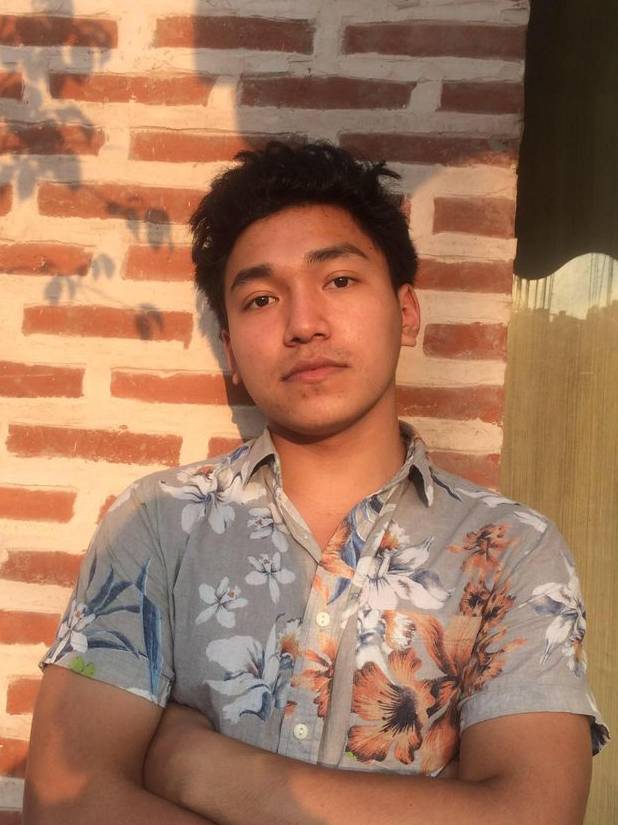
HTML tags are like labels that tell the browser what each part of a webpage is.
For example, if you want to show a heading, you use a heading tag like this:
Apples
The tag tells the browser that the text
Apple
is a heading and it should be big and bold.
Tags usually come in pairs: an opening tag like and a closing tag like
. Whatever you put between them is the content.
Tags not visible on the page—just instructions for how to show things. Only the content is visible.
As you continue with the course, you'll learn plenty of tags, each with their own purpose.
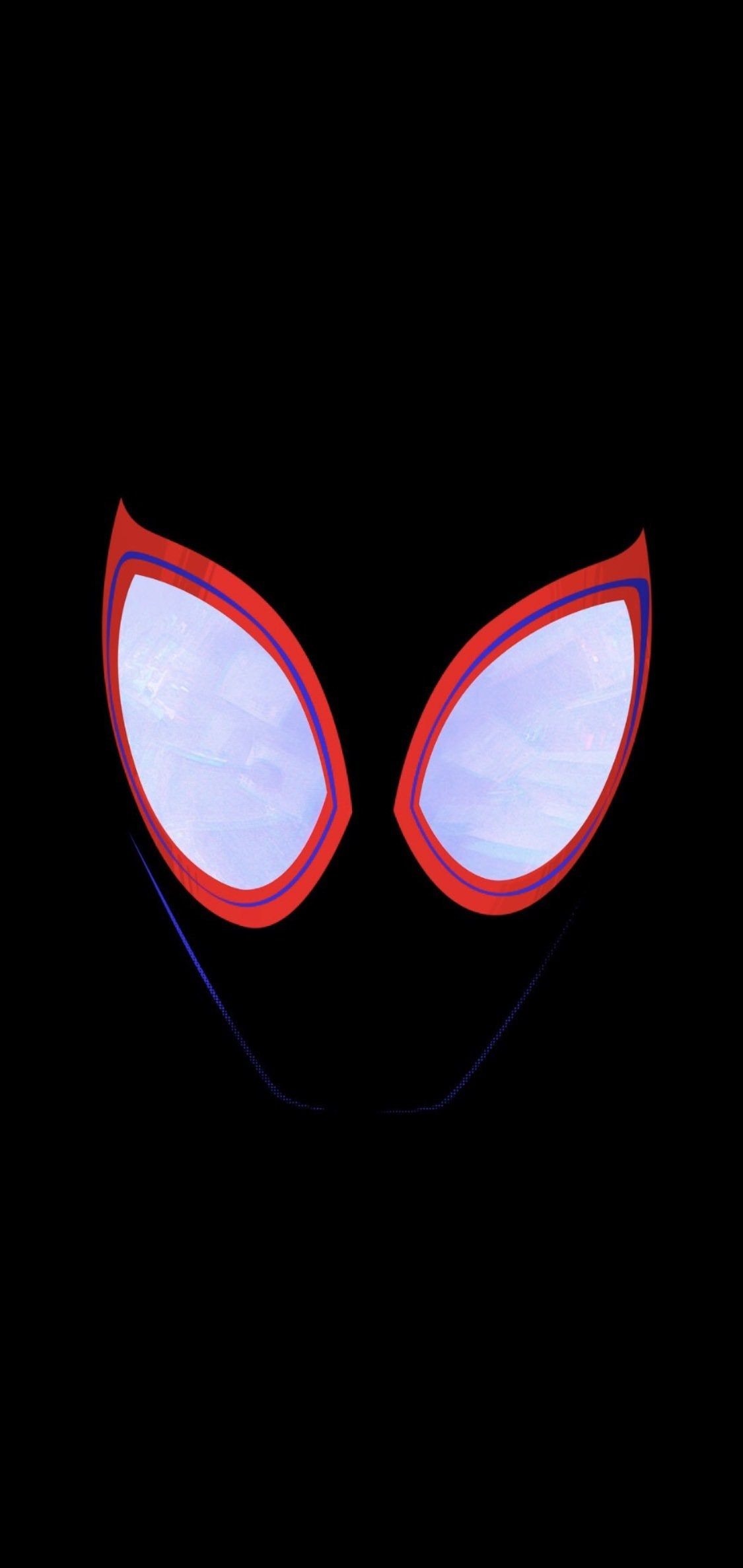
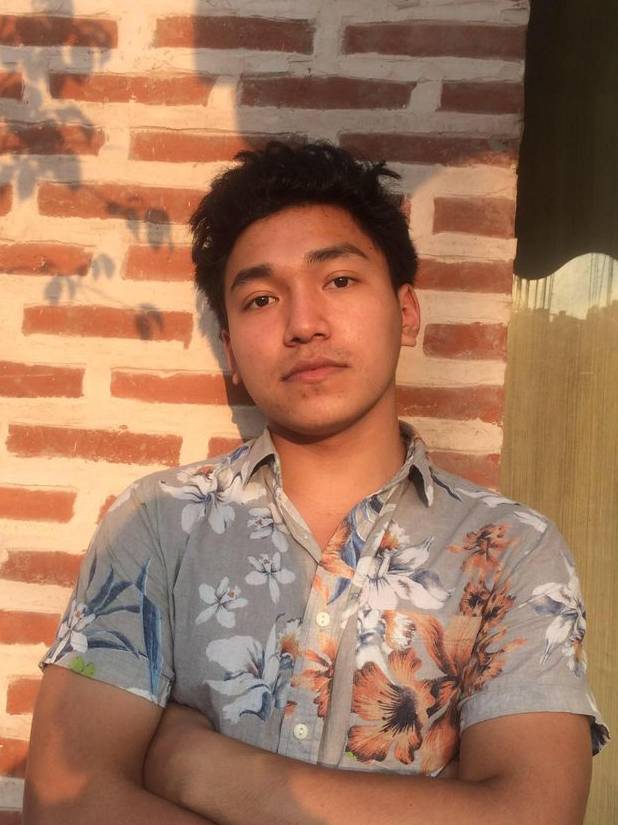
The key thing to note is that lists, tuples, and dictionaries are simply different ways to store data. Which one you choose depends on what you need your program to do.
That said, here are the major differences between them:
List: An ordered collection that can be changed (mutable).
my_list = [1, 2, 3]
my_list[0] = 10 # You can change items
print(my_list) # Output: [10, 2, 3]
Tuple: Also ordered, but cannot be changed (immutable).
my_tuple = (1, 2, 3)
# my_tuple[0] = 10 # This would cause an error
print(my_tuple) # Output: (1, 2, 3)
Dictionary: Stores data as key-value pairs, and you access values using keys.
my_dict = {"name": "Ali", "age": 25}
print(my_dict["name"]) # Output: Ali
my_dict["age"] = 26 # You can update values
So, lists and tuples store values by position — but only lists can be changed. Dictionaries store data using keys, which makes them great when you want to label and quickly access your data.
Let me know if you need more clarification on this.
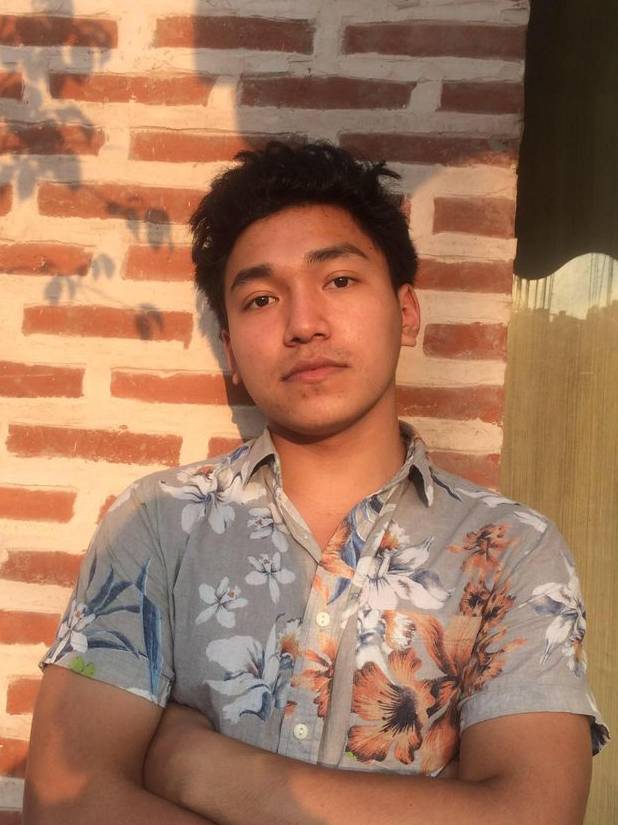
The purpose of printing output — like using cout
in C++ — is to display something on the screen so you can see what your program is doing.
It helps you understand if your code is working as expected, especially when you're learning. Think of it as a way for your program to "talk" to you.