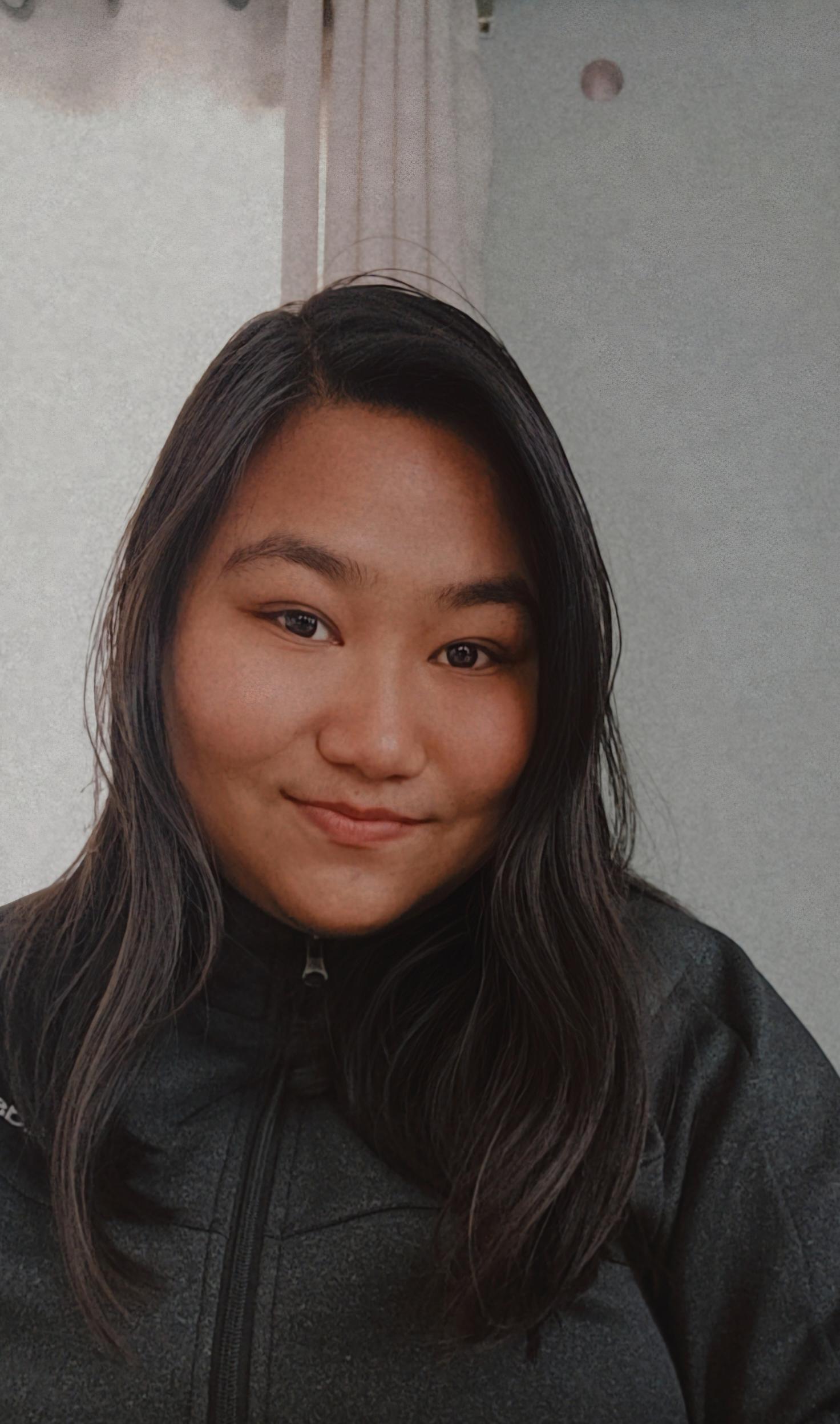
Palistha Singh
Technical Content Writer @Programiz
Answered 5 questions
About
Hey there! I'm Palistha, Technical Content Writer at Programiz. My goal is to make programming easy and fun for everyone, whether you're just starting out or looking to expand your skills. I believe learning should be interactive, so I'm here to help you and make your learning experience smooth and enjoyable. Let's learn and grow together!
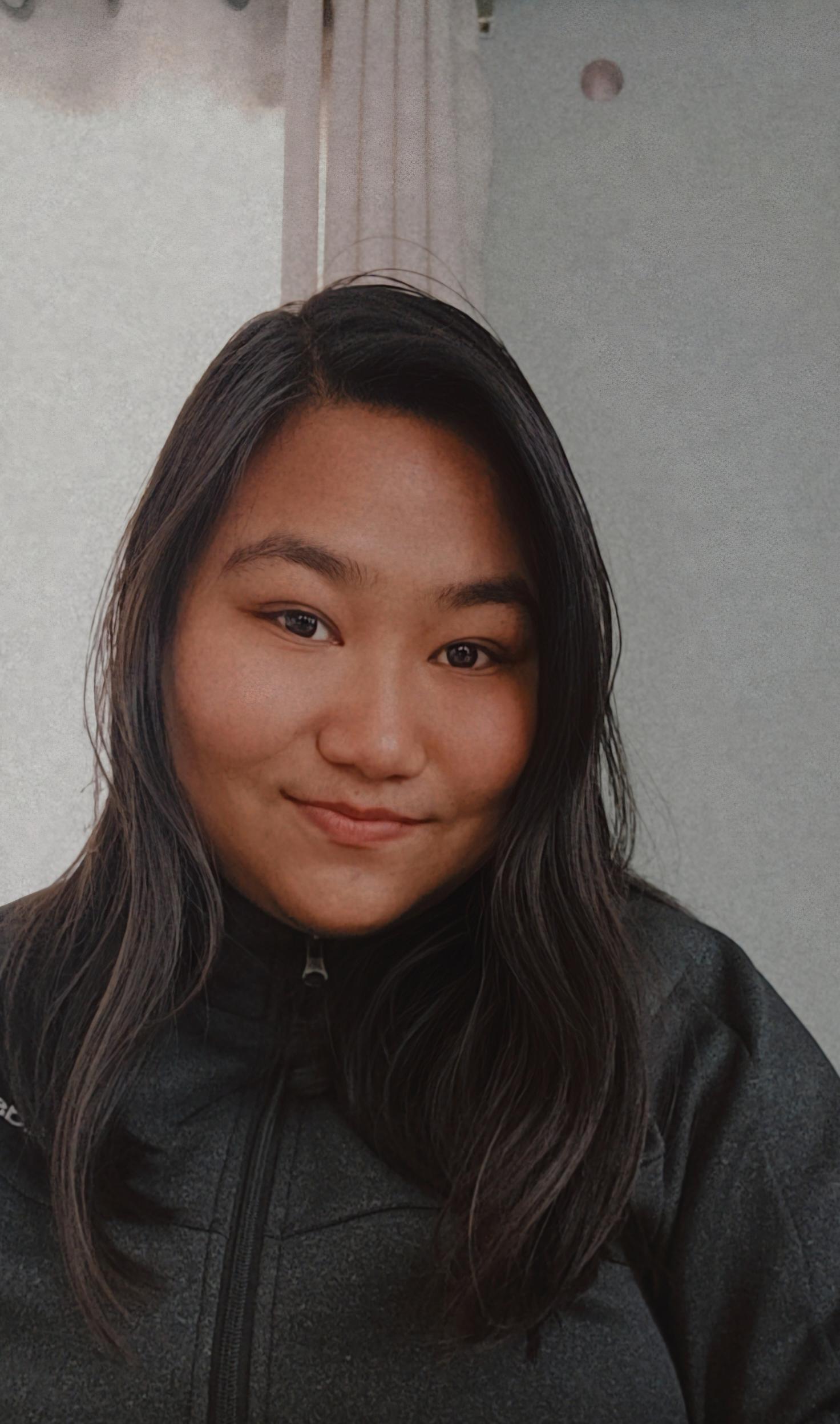
Yes, there’s an important difference between public class
and just class
in Java. Here’s what you need to know:
1. Accessibility Difference
public class
: The class can be accessed from anywhere in your program (even from other packages/folders).
public class Main { // Can be used everywhere
public static void main(String[] args) {
System.out.println("Hello!");
}
}
class
(no modifier): The class is only accessible within its own package/folder.
class Helper { // Only usable in this package
void doSomething() { ... }
}
2. File Naming Rule (For public Classes)
If you declare a class as public
, the filename must match the class name.
Name of the file containing
public class Main { ... }
should be Main.java.Name of the file containing
public class MyProgram { ... }
can't be Main.java.
3. When to Use Which?
Use
public
for classes that need to be shared across your project (likeMain
).Use default (
class
withoutpublic
) for helper classes that only one package uses.
// File: Main.java (Public, so filename matches)
public class Main {
public static void main(String[] args) {
Helper.help(); // Can only call if Helper is public or in same package
}
}
// File: Helper.java (No 'public' = package-private)
class Helper {
static void help() {
System.out.println("Assisting...");
}
}
Key Takeaway:
public
means anyone can use this class.No modifier means only my package/folder can use this class.
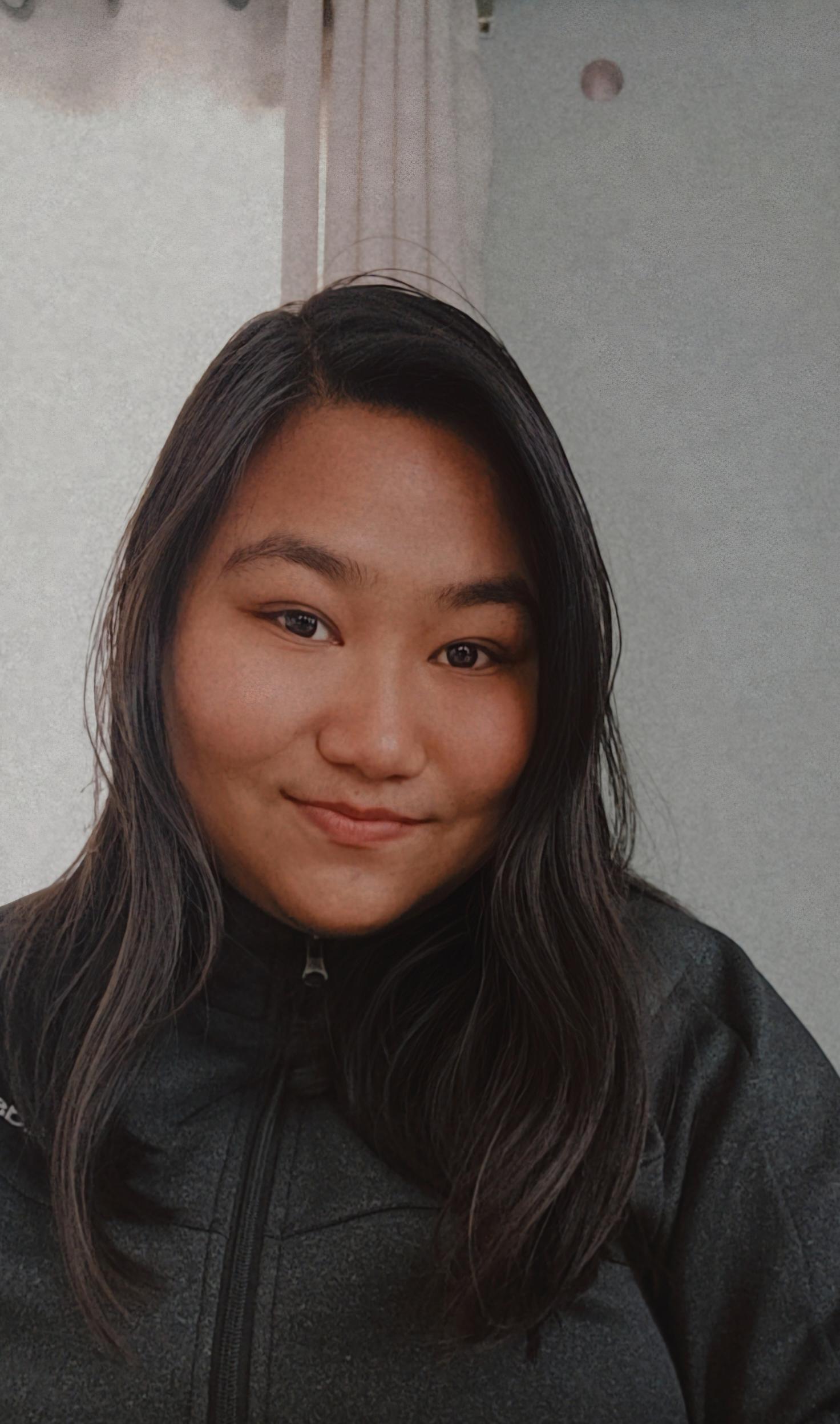
That's a good question!
When you look at the output, it can be difficult to notice extra spaces. That's why it's better to check the code carefully.
For example:
print("godfrey sami")
Here, there are no extra spaces before or after the text.
But:
print(" godfrey sami ")
In this case, there are spaces before and after the text — and you can clearly see that by looking at the code.
There are other ways to check for spaces automatically, but for now, we don't want to overwhelm you.
Just click on Next Lesson to keep going!
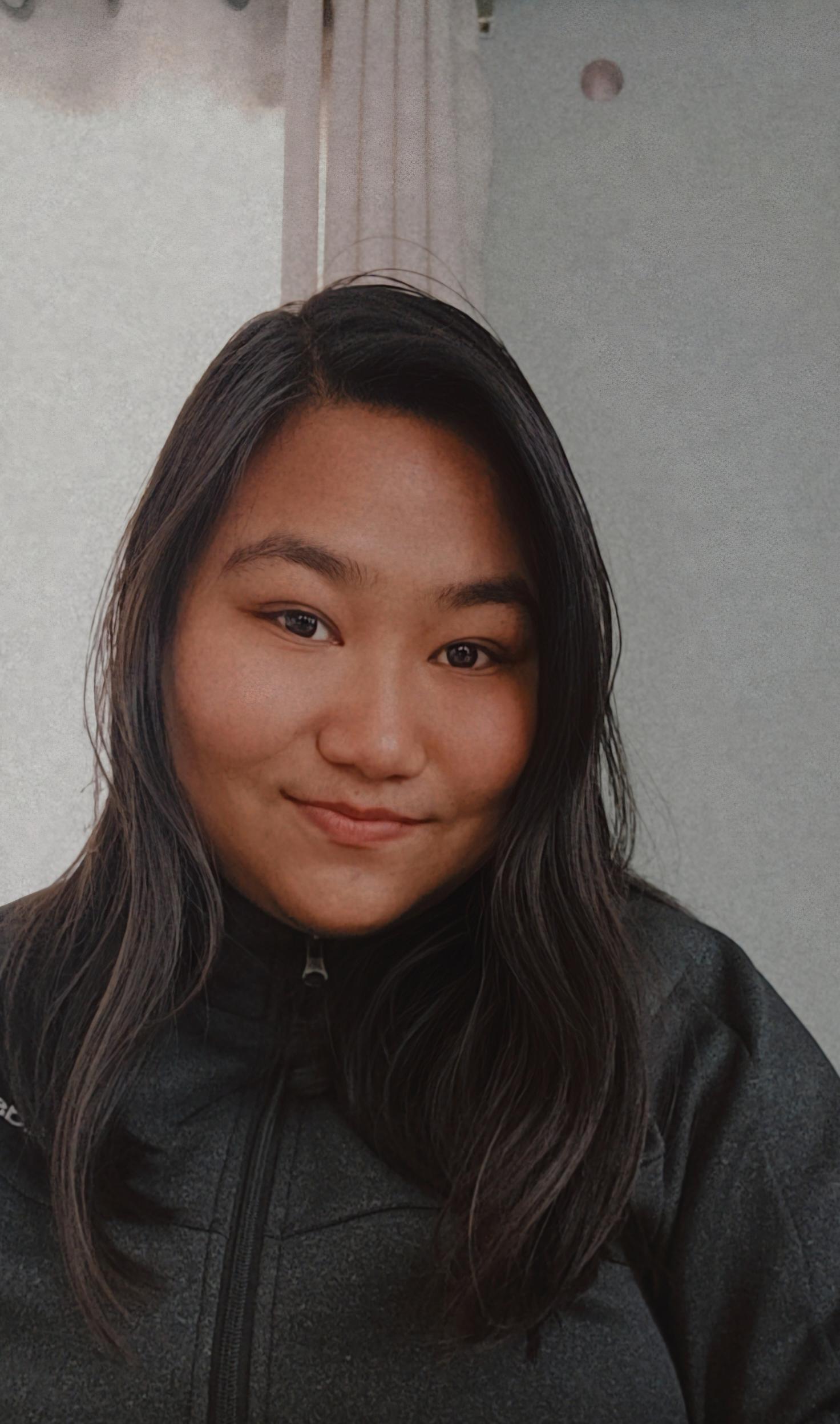
That's a great question!
Working with SQL is different from entering data into Excel because, with SQL, you don't manually type or organize the data yourself.
Instead, the data is already stored in a database, and you use SQL commands to find, retrieve, organize, and analyze that data automatically.
In Excel, you often work with small amounts of data by hand. In SQL, you can work with huge amounts of data much faster and more efficiently using simple queries.
If you have more questions, I'm here to help!
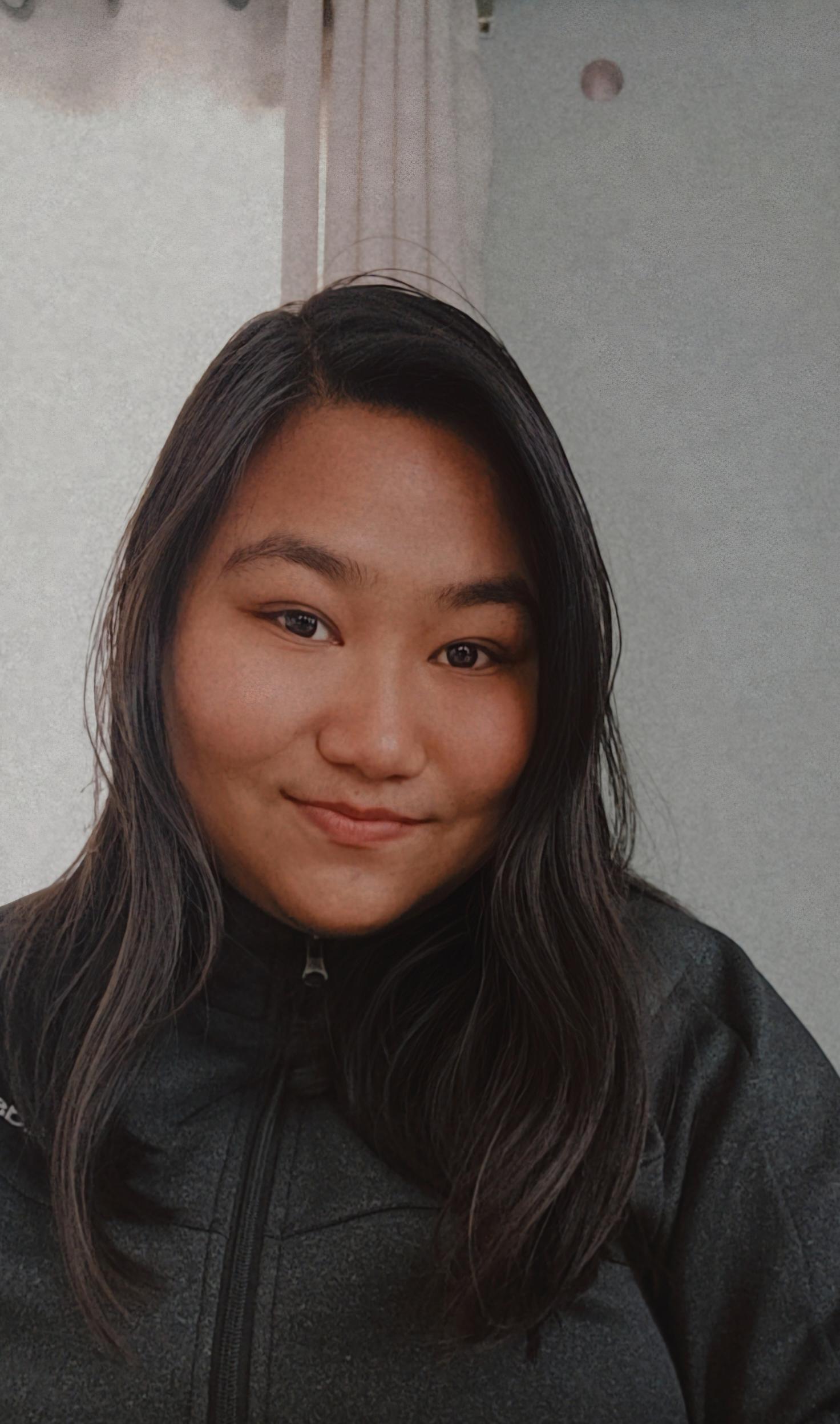
In Python, multi-line strings are used when you want to store or display text that spans across multiple lines — instead of just one.
You define a multi-line string using triple quotes ('''
or """
).
Example:
message = """Hello!
Welcome to Python programming.
This is a multi-line string."""
print(message)
Output:
Hello!
Welcome to Python programming.
This is a multi-line string.
If you have more questions, I’m here to help — feel free to ask anytime!
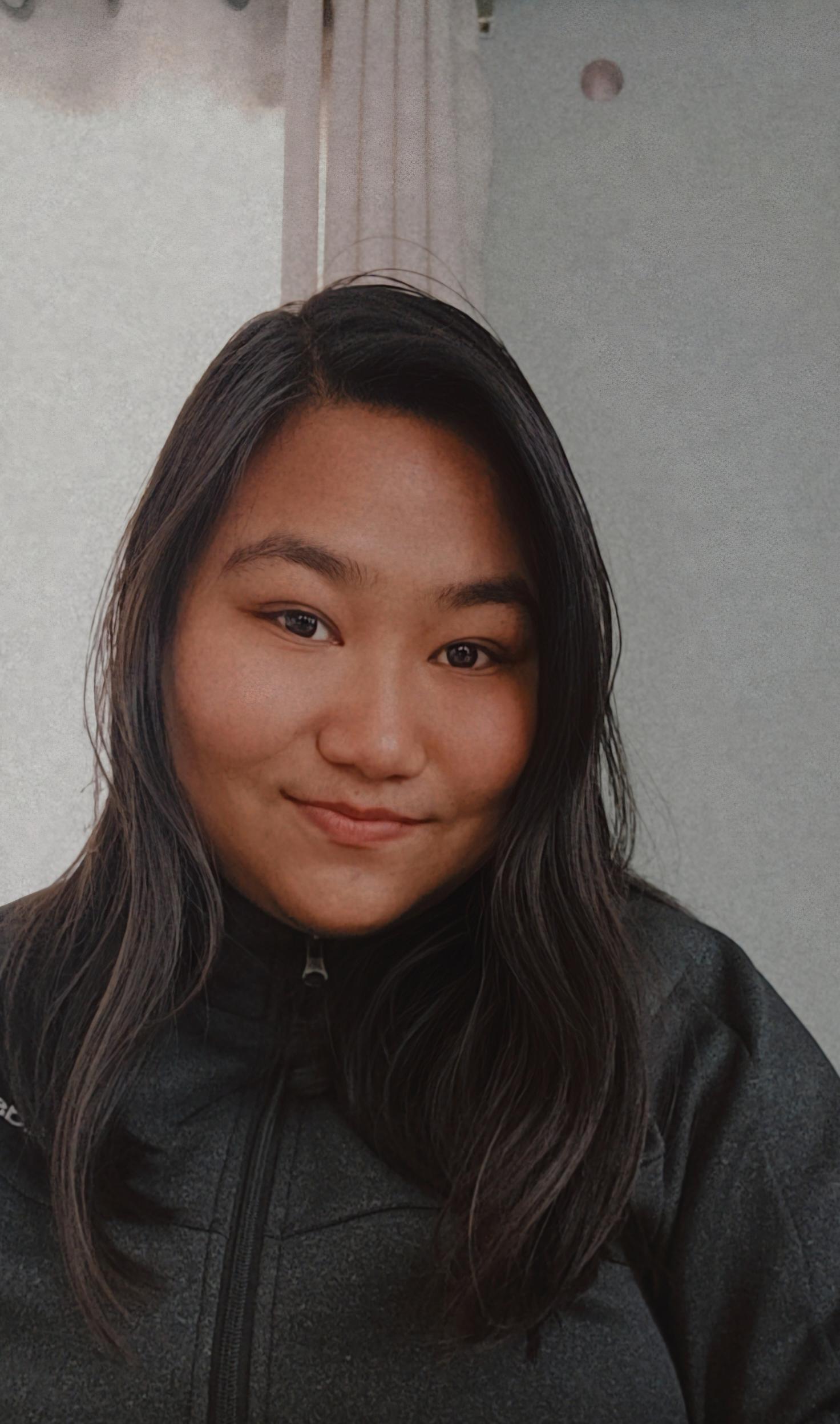
endl
in C++ stands for "end line". It's used to move the output to the next line, just like pressing Enter on the keyboard.
For example:
cout << number1 << endl;
This prints the value of number1
, then moves the cursor to the next line before printing anything else.
We can clearly see this in the practice exercise:
int number1 = 89;
int number2 = 313.78;
cout << number1 << endl;
cout << number2;
Output with endl
:
89
313.78
Without endl
(if we wrote cout << number1;
):
89313.78
So endl
helps keep output clean and readable by separating it into lines.