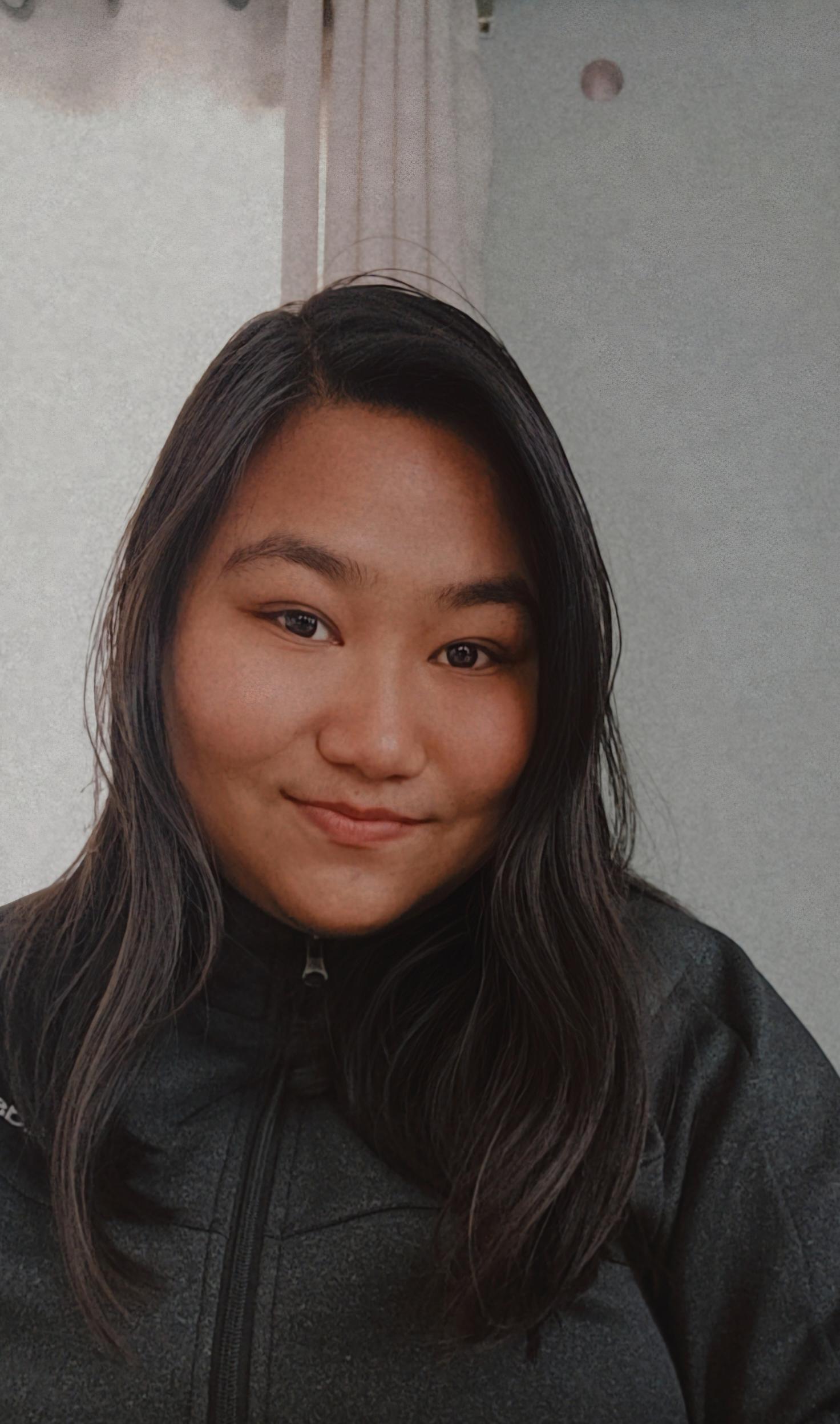
Yes, there’s an important difference between public class
and just class
in Java. Here’s what you need to know:
1. Accessibility Difference
public class
: The class can be accessed from anywhere in your program (even from other packages/folders).
public class Main { // Can be used everywhere
public static void main(String[] args) {
System.out.println("Hello!");
}
}
class
(no modifier): The class is only accessible within its own package/folder.
class Helper { // Only usable in this package
void doSomething() { ... }
}
2. File Naming Rule (For public Classes)
If you declare a class as public
, the filename must match the class name.
Name of the file containing
public class Main { ... }
should be Main.java.Name of the file containing
public class MyProgram { ... }
can't be Main.java.
3. When to Use Which?
Use
public
for classes that need to be shared across your project (likeMain
).Use default (
class
withoutpublic
) for helper classes that only one package uses.
// File: Main.java (Public, so filename matches)
public class Main {
public static void main(String[] args) {
Helper.help(); // Can only call if Helper is public or in same package
}
}
// File: Helper.java (No 'public' = package-private)
class Helper {
static void help() {
System.out.println("Assisting...");
}
}
Key Takeaway:
public
means anyone can use this class.No modifier means only my package/folder can use this class.