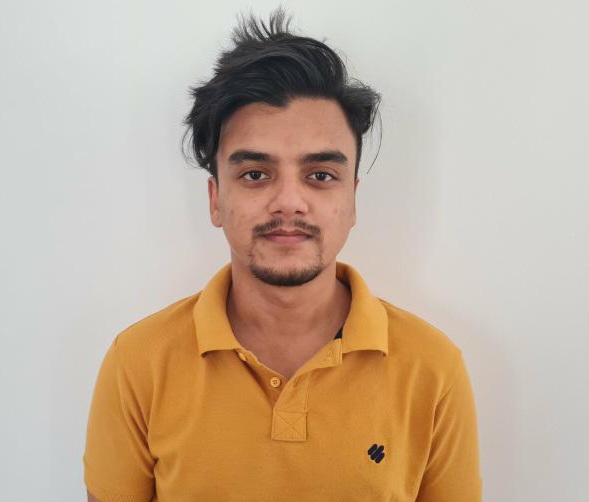
Let me try to explain the concept.
A number is called prime if it is only divisible by 1 and itself. If no other number can divide it exactly, then the number is prime.
So to check if a number is prime, we try dividing it by all numbers between 2 and (number - 1).
If it divides evenly by any of them, it's not a prime number.
If it doesn’t divide evenly by any, then it's prime.
Here’s a function that checks if a number is prime:
void isPrime(int number) {
int flag = 0;
for (int i = 2; i < number; ++i) {
if (number % i == 0) {
flag = 1;
}
}
if (flag == 0) {
printf("%d\n", number);
}
}
Here,
We run a loop from
2
tonumber - 1
.In each iteration, we check if
number
is divisible byi
.If it is divisible, we set
flag
to1
(meaning it’s not prime).After the loop, if
flag
is still0
, it means the number is prime, and we print it.
Then, in the main()
function, we can call isPrime()
for a range of numbers:
int main() {
int x, y;
scanf("%d %d", &x, &y);
// run the loop from x to y
// for each iteration of loop call isPrime()
for (int i = x; i <= y; ++i) {
isPrime(i);
}
return 0;
}
Hope this helps and makes the concept clearer! Feel free to reach out if you have more questions or need further assistance.