PRO
Izzy
asked
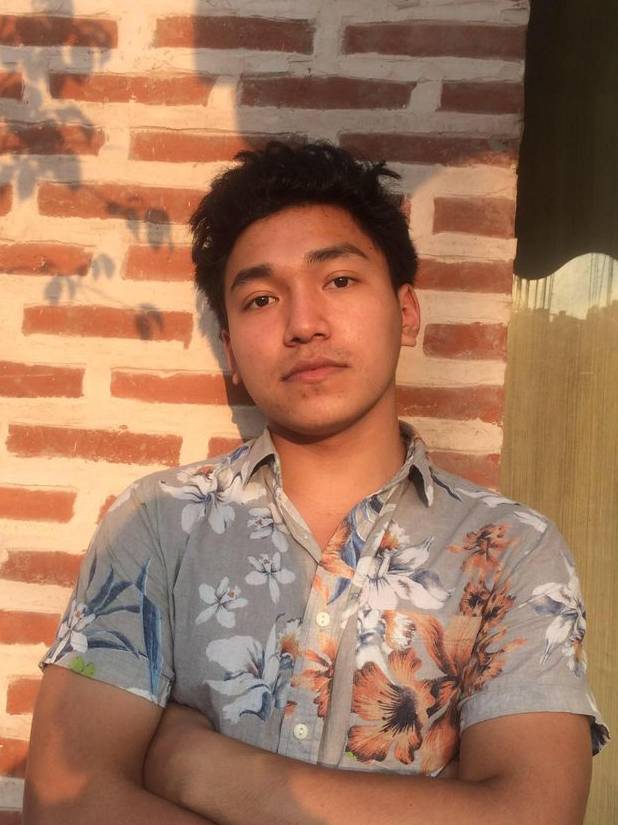
Expert
Kelish Rai answered
The difference between ++i
and i++
comes down to when the increment happens.
++i
(pre-increment) increases the value ofi
first, then uses it.i++
(post-increment) uses the current value ofi
first, then increases it.
Here's a simple example to illustrate:
#include
int main() {
int i = 5;
printf("Initial value: %d\n", i); // Output: 5
// Post-increment: Uses i first, then increments
printf("Post-increment (i++): %d\n", i++); // Output: 5
printf("After post-increment, i = %d\n", i); // Output: 6
// Reset i
i = 5;
// Pre-increment: Increments i first, then uses it
printf("Pre-increment (++i): %d\n", ++i); // Output: 6
printf("After pre-increment, i = %d\n", i); // Output: 6
return 0;
}
Note:
If you just need to increase a value and don't care when it happens (like in a simple loop), it usually doesn't matter which one you use.
But if you are using the value immediately in an expression (like passing it to a function or using it in a calculation), whether you use pre-increment or post-increment can change the result.
C
This question was asked as part of the Learn C Programming course.