PRO
Oshin
asked
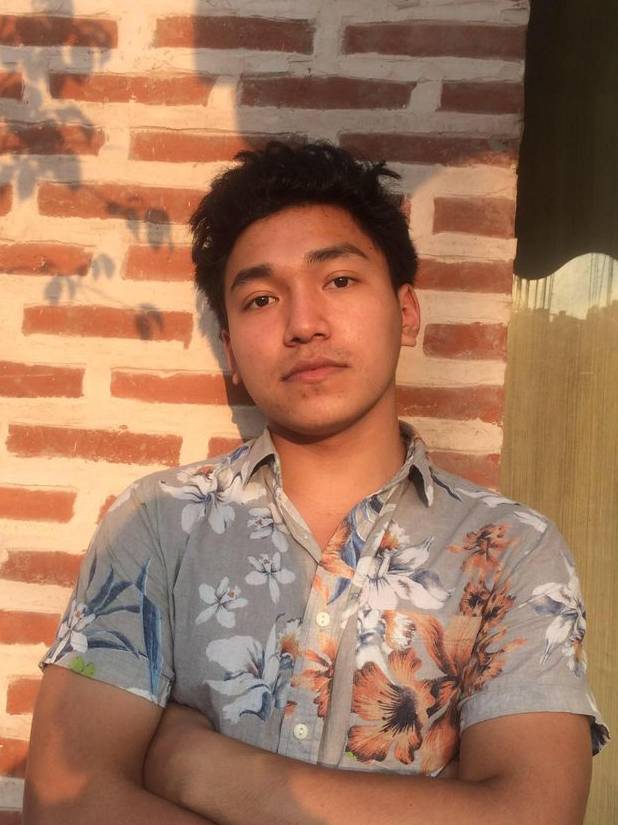
Expert
Kelish Rai answered
That's alright. Let me break it down simply.
When writing programs, we often need to take input from the user. For example, if we want to ask for a name and then greet the user, we need a way to get that input.
In C++, we use cin
to take input and store it in a variable. Here’s how it works:
#include
using namespace std;
int main() {
string name;
cout << "Enter your name: ";
// Take user's name as input
// Store user's name in name variable
cin >> name;
// Greet user
cout << "Hello, " << name;
return 0;
}
If you run this program, you'll get this as output:
Enter your name:
Now, you need to click on the output and enter your name. Suppose you enter John
:
Enter you name: John
Now, cin >> name;
stores "John"
in the name
variable.
Finally, cout << "Hello, " << name;
prints:
Hello, John
The thing to note here is that you need to use >>
when using cin
and <<
when using cout
. Otherwise, you may get an error.
C++
This question was asked as part of the Learn C++ Basics course.