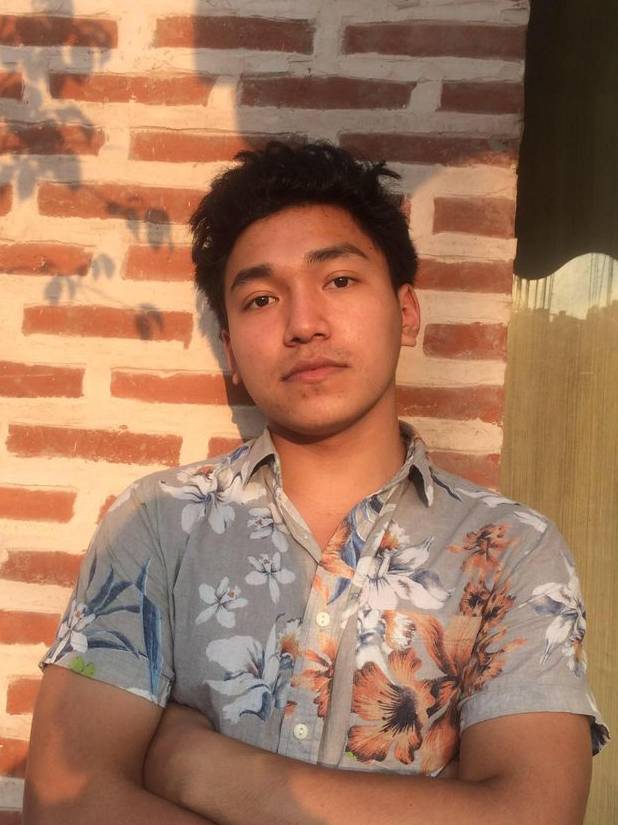
The main difference between arrays and vectors is that an array is a fixed-size collection of elements, whereas a vector can grow or shrink as needed.
Both are used to store multiple values, but they work a bit differently.
With arrays, once you define the size, it can't be changed. For example:
int arr[5];
Here, you've got space for exactly 5 integers—no more, no less. If you later want to store 6 or more values, you'll need to create a new array and manage copying manually.
Vectors, on the other hand, are part of the C++ Standard Library and are more flexible. You can start with an empty vector and keep adding elements using push_back()
:
#include
using namespace std;
vector nums; // Starts empty
nums.push_back(10); // Adds 10 to the vector
nums.push_back(20); // Adds 20
The vector automatically resizes itself in the background as you add elements—no need to specify the size in advance.
Note: If you're working with a known, fixed number of values and performance is critical, arrays can be slightly faster. But in most cases, vectors are preferred for their ease of use and flexibility.