PRO
Sebastian
asked
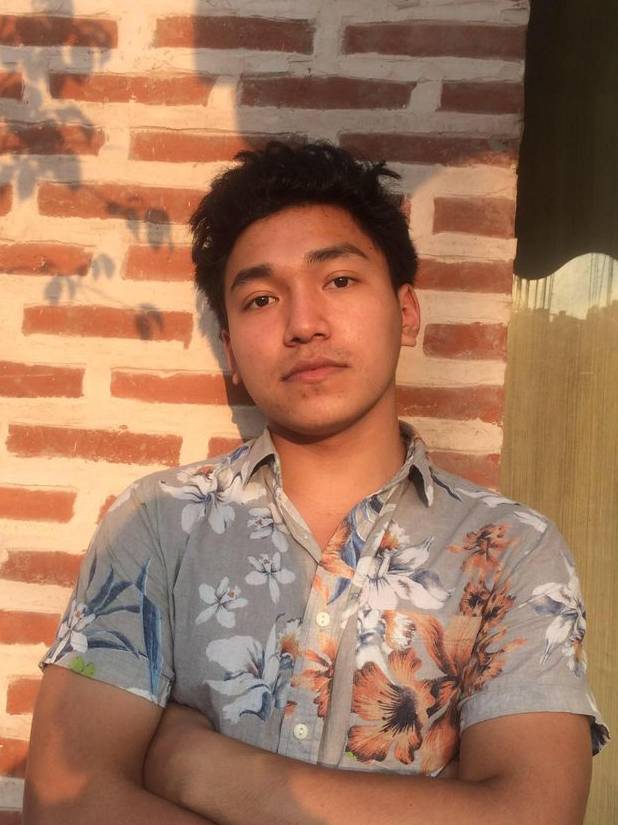
Expert
Kelish Rai answered
In the code:
#include
using namespace std;
int main() {
// create a variable
double number = 83.13;
// create a pointer variable
double* pt;
// assign address to pointer
pt = &number;
// print pointer pt
cout << pt;
return 0;
}
The reason the pointer needs to be a double*
is that the variable number
is of type double
.
In C++, the type of a pointer should always match the type of the variable it points to. So if number
were an int
, then the pointer should also be an int*
.
Here's a quick comparison to help make it clearer:
int a = 5;
int* ptr1 = &a; // OK: both are int
double b = 6.2;
double* ptr2 = &b; // OK: both are double
double* wrongPtr = &a; // Not OK: types don’t match (int vs double)
Using the correct pointer type ensures that the program knows how much memory to access and interpret properly. For instance, a double
typically uses more bytes than an int
, and a mismatch can lead to unexpected behavior.
C++
This question was asked as part of the Learn C++ Basics course.