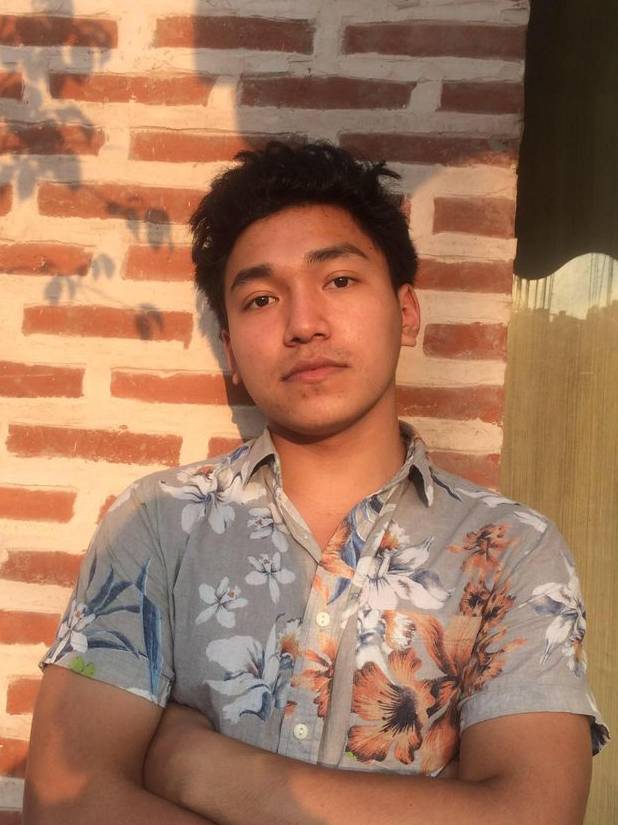
You're absolutely right that both else if
and
switch
can be used to handle multiple conditions, and it often
comes down to personal preference or the situation.
However, there are a few advantages to using switch
:
-
Cleaner code: When you're comparing a single variable to many possible values,
switch
often results in cleaner and more readable code than multipleelse if
statements. -
Efficiency: In some cases,
switch
can be more efficient. When you have many conditions to check for the same variable,switch
can sometimes make the process faster than evaluating each condition one by one aselse if
does.
Here’s a simple example in C++ to show the difference:
// Using else if
#include
using namespace std;
int main() {
int day = 3;
if (day == 1) {
cout << "Monday";
} else if (day == 2) {
cout << "Tuesday";
} else if (day == 3) {
cout << "Wednesday";
} else {
cout << "Another day";
}
return 0;
}
In this example, each condition is checked one by one until a match is found.
// Using switch
#include
using namespace std;
int main() {
int day = 3;
switch(day) {
case 1:
cout << "Monday";
break;
case 2:
cout << "Tuesday";
break;
case 3:
cout << "Wednesday";
break;
default:
cout << "Another day";
}
return 0;
}
Here, the switch
allows the program to directly jump to the
matching case
block, which can be more efficient and helps the
logic feel more organized.
Both approaches work well, and it’s good to be comfortable with both. When
dealing with a single variable and multiple constant values,
switch
tends to be a cleaner and often faster choice.