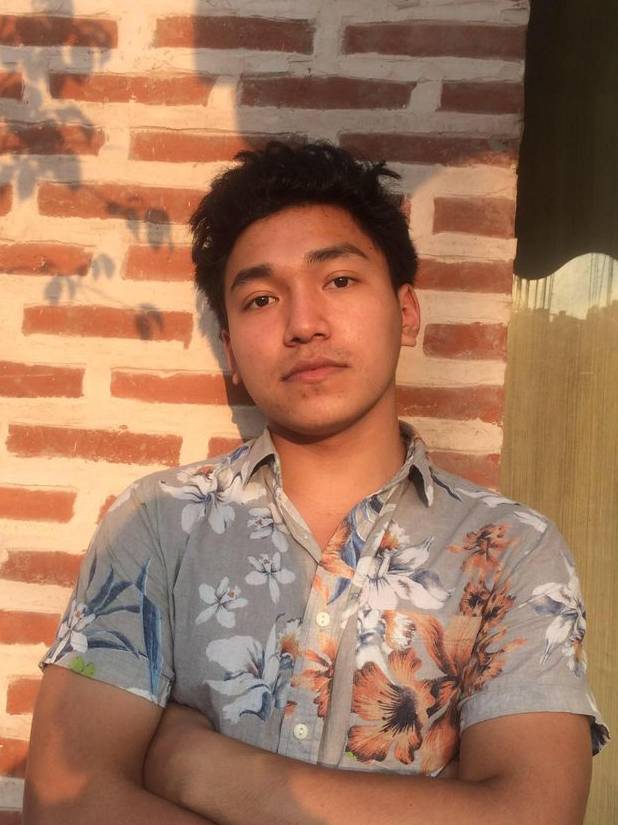
The break
statement is used to stop a loop based on certain conditions. It can be used to terminate any kind of loop—for
, while
, etc.
Here’s an example:
class Main {
public static void main(String[] args) {
for (int i = 1; i <= 10; i++) {
System.out.println("Hello Dhanush");
break;
}
}
}
Output
Hello Dhanush
According to the for
loop’s condition (i <= 10
), the loop is supposed to run 10
times. But because we’ve used break
right after the first print statement, the loop stops immediately after the first iteration.
Now let’s say we want to stop the loop after 5
iterations instead of immediately:
class Main {
public static void main(String[] args) {
for (int i = 1; i <= 10; i++) {
System.out.println("Hello Dhanush");
if (i == 5) {
break;
}
}
}
}
Output
Hello Dhanush
Hello Dhanush
Hello Dhanush
Hello Dhanush
Hello Dhanush
Here, Hello Dhanush
is printed 5
times. That’s because we’ve used the condition if (i == 5)
to break out of the loop when i
reaches 5
.
Note: break
is very useful when you want to exit a loop early, without having to wait for the loop's natural condition to end. You can apply this same logic in different scenarios, like stopping a search when the target is found.