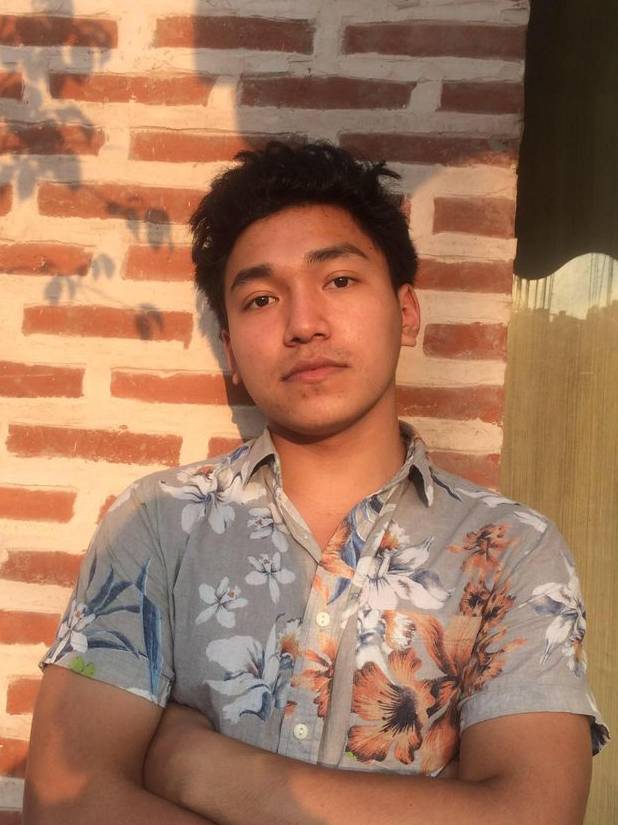
A HashMap
is not ordered in Java. It doesn’t guarantee any specific order of elements when you add or retrieve them. The reason for this is that HashMap
is optimized for fast lookups, and ordering elements doesn’t contribute to its primary goal.
If you need an ordered version, you have two options:
1. LinkedHashMap
: This data structure maintains the order of insertion. That means the order in which you add elements to the map will be preserved when you iterate over it.
Example:
Map linkedMap = new LinkedHashMap<>();
linkedMap.put("one", 1);
linkedMap.put("two", 2);
linkedMap.put("three", 3);
// Output will be in insertion order
for (Map.Entry entry : linkedMap.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
2. TreeMap
: This data structure automatically sorts the keys in natural order (or by a custom comparator if provided). The elements will be sorted based on the key.
Example:
Map treeMap = new TreeMap<>();
treeMap.put("banana", 2);
treeMap.put("apple", 1);
treeMap.put("cherry", 3);
// Output will be in sorted order by key
for (Map.Entry entry : treeMap.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
So, if you want ordered behavior, you’d use either LinkedHashMap
for insertion order or TreeMap
for sorted order.