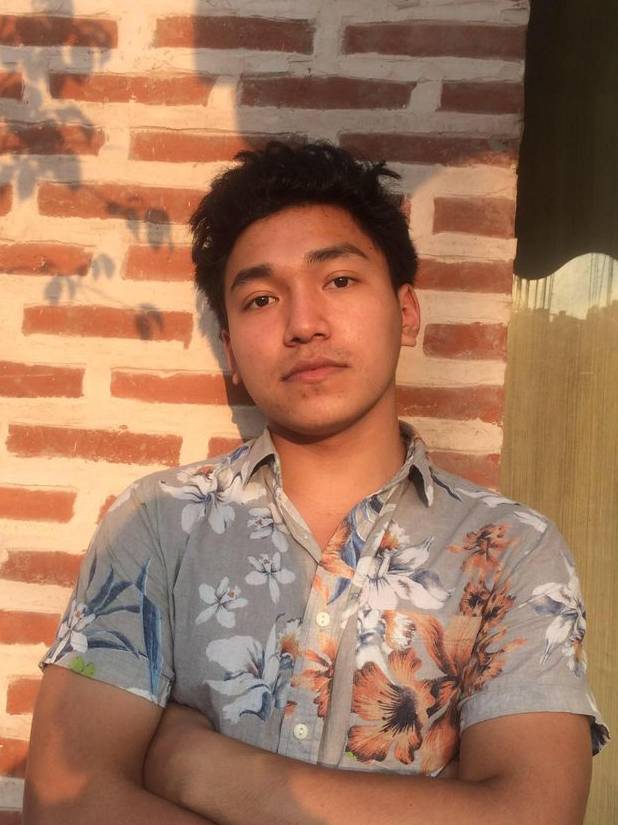
You're right—using if
vs elif
isn't drastically different in many cases. You can even use multiple if
statements instead of elif
to check several conditions.
However, it's important to understand how Python reads and handles if...elif...else
compared to a series of if...if...if
.
When you use if...elif...else
, Python stops checking as soon as it finds a condition that's true. On the other hand, if you use multiple if
statements, Python will check each one separately, even if an earlier one was already true.
Here's a quick example to show the difference:
1. Using if...elif...else:
x = 10
if x > 5:
print("Greater than 5")
elif x > 3:
print("Greater than 3")
elif x > 7:
print("Greater than 7")
Output:
Greater than 5
Here, only the first block runs because its condition is true
.
2. Using if...if...if:
x = 10
if x > 5:
print("Greater than 5")
if x > 3:
print("Greater than 3")
if x > 7:
print("Greater than 7")
Output:
Greater than 5
Greater than 3
Greater than 7
Here, all conditions are checked separately, so all print statements are executed.
Also, it’s worth noting that using multiple if
statements is quite common in real programs, especially when you're checking conditions that aren't mutually exclusive. You'll be using them a lot as you build more complex logic.