Ask Programiz - Human Support for Coding Beginners
Explore questions from fellow beginners answered by our human experts. Have some doubts about coding fundamentals? Enroll in our course and get personalized help right within your lessons.
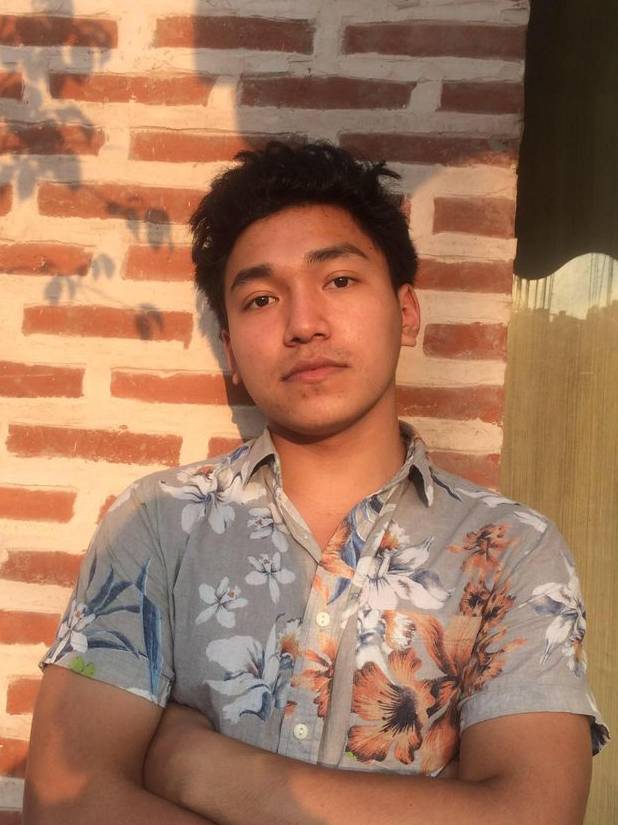
Yes, console.log()
in JavaScript is similar to print()
in Python — both are used to display information to the console or terminal.
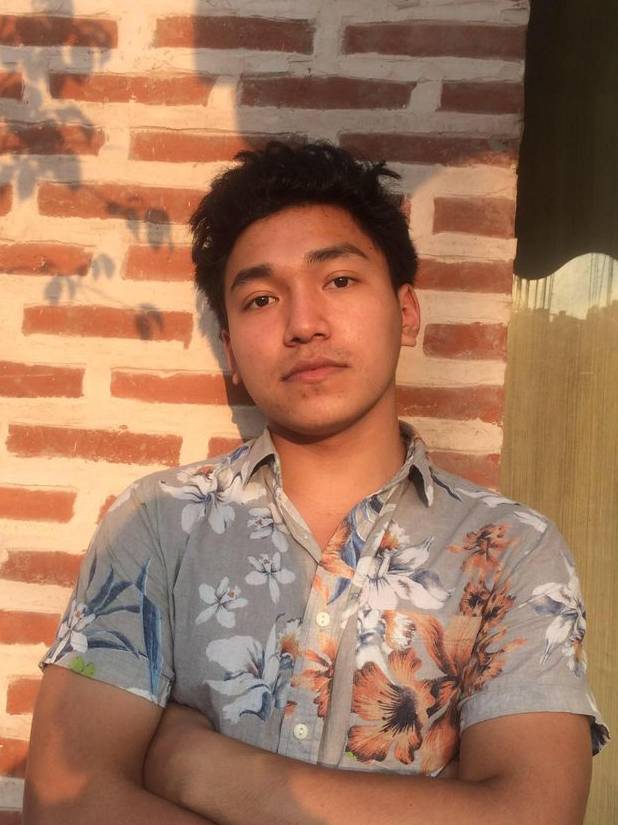
The difference between innerHTML
and outerHTML
is in what part of the element they work with.
innerHTML
gives you just the content inside an element.outerHTML
gives you the entire element itself, including the tag.
Consider this code:
Hello
And this JavaScript:
const hero = document.querySelector(".demo");
Now if you do:
console.log(hero.innerHTML);
You'll get:
Hello
But if you do:
console.log(hero.outerHTML);
You'll get:
Hello
So, outerHTML
includes the entire element, while innerHTML
only includes what's inside it.
Simply put, use innerHTML
if you want to change the content inside an element, and use outerHTML
if you want to change the element itself.
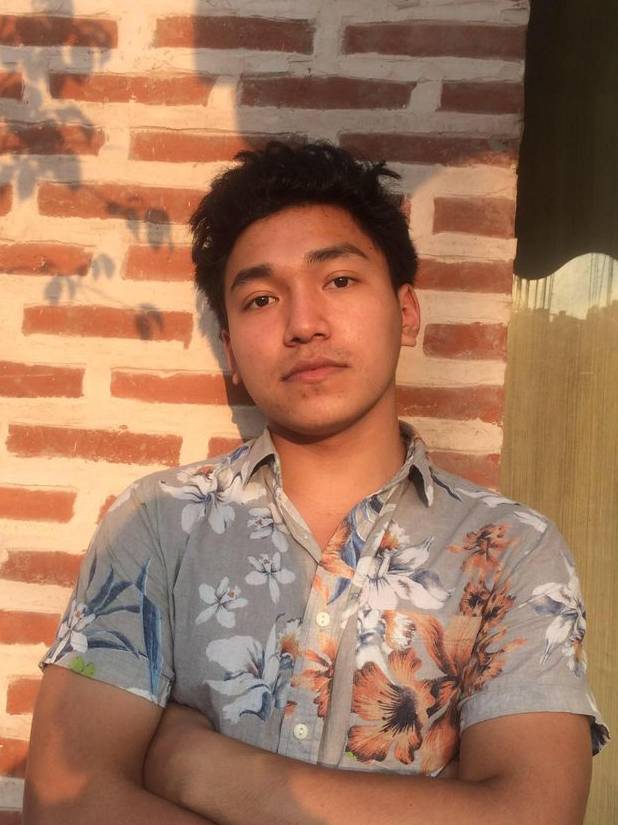
In JavaScript, variable names cannot start with a number. They need to begin with a letter, an underscore (_
), or a dollar sign ($
). However, you can use numbers in the middle or at the end of the variable name.
For example, these are valid variable names:
myVariable1
variable_2
$_value
But these are not valid:
1stVariable
123name
Note: If you try to start a variable name with a number, JavaScript will throw a syntax error when you run the code.
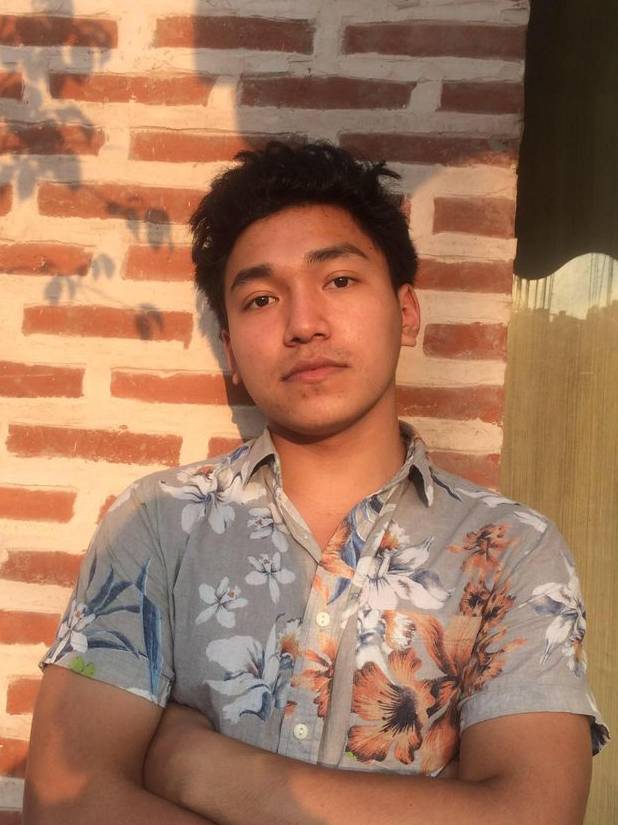
It's not mandatory to know HTML or CSS before getting started with JavaScript.
JavaScript is a versatile language — it’s used for everything from making web pages interactive to building servers, mobile apps, and even games. So if you're learning it for general programming purposes (like game development or backend work), you can absolutely start without knowing any HTML or CSS.
That said, if your goal is to build websites or web applications, having a basic understanding of HTML and CSS will definitely make things easier.
HTML provides the structure of a webpage (like headings, paragraphs, images).
CSS handles the styling (like colors, fonts, layouts).
JavaScript adds interactivity (like responding to clicks, form submissions, animations).
Since JavaScript often works closely with HTML and CSS in web development, knowing a little bit about them will help you understand where and how to apply JavaScript effectively.
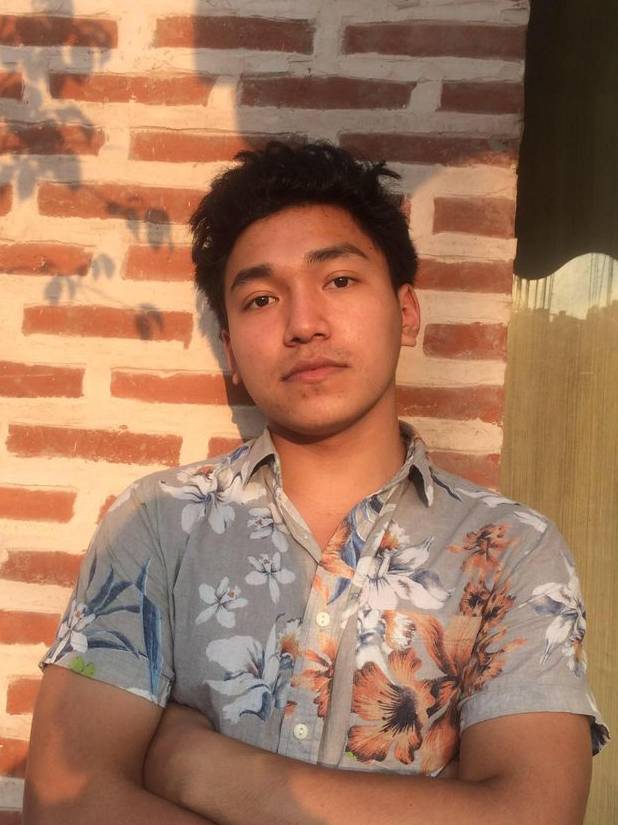
Arrays work by storing multiple values in a single variable. Instead of creating a new variable for every value, you can group related values together in an array. This is especially useful when you have a list of items.
Here's a simple example:
const fruits = ["apple", "banana", "orange"];
In this example, fruits
is an array that holds three values: apple
, banana
, and orange
.
Here are some things that you can do with arrays:
Access values using their index:
fruits[0]
Update values:
fruits[1] = "mango"
Add values:
fruits.push("grape")
Loop through values
Arrays are a basic but powerful tool in programming, and you’ll use them often when working with lists, collections, or structured data.