Ask Programiz - Human Support for Coding Beginners
Explore questions from fellow beginners answered by our human experts. Have some doubts about coding fundamentals? Enroll in our course and get personalized help right within your lessons.
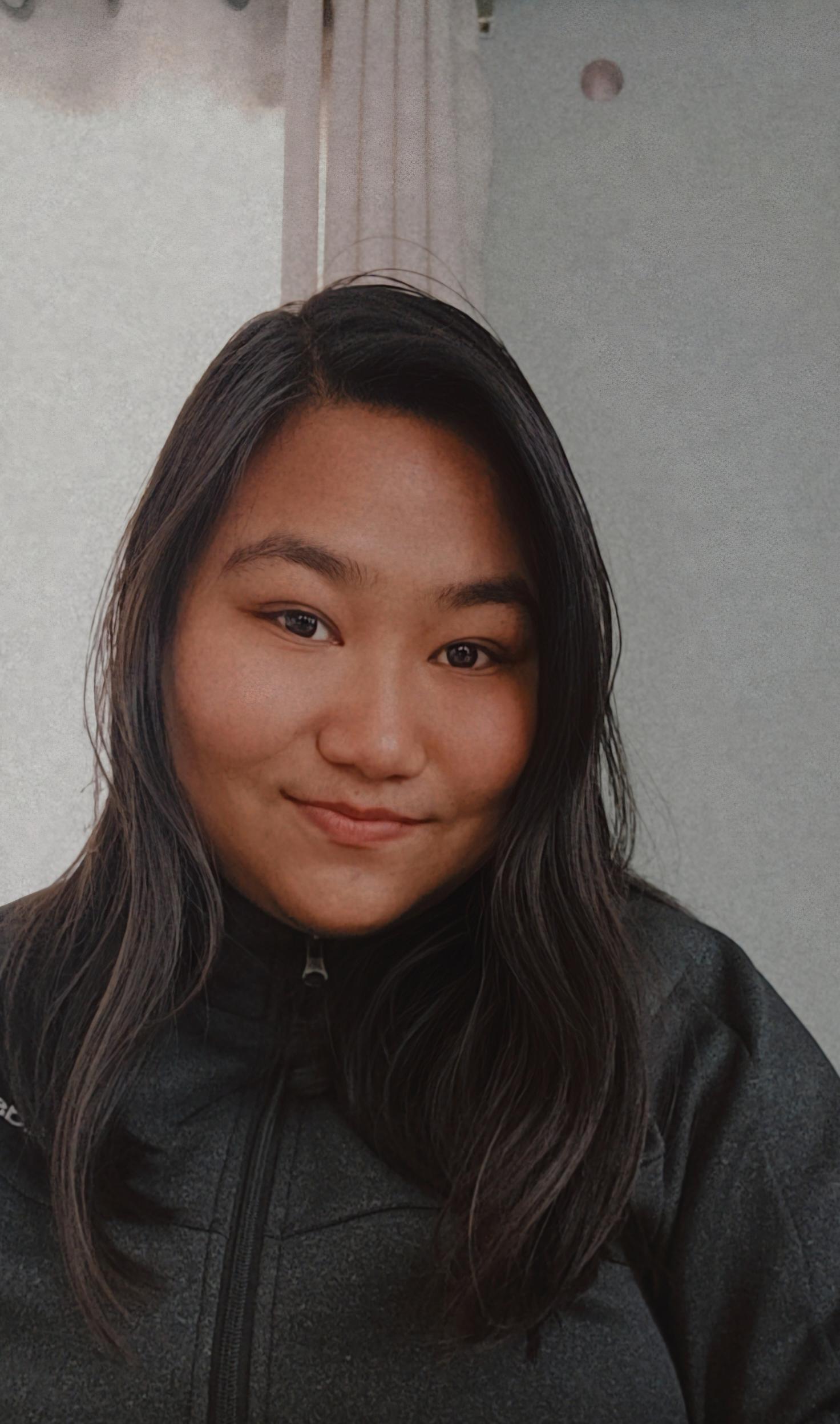
Hey! In programming, fixed values are values you write directly into your code — they don't change while the program runs. These are called literals.
For example:
5
→ an integer literal3.14
→ a floating-point (double) literal"Hello"
→ a string literal
They're called "fixed" because you're using them directly in your code, without assigning them to variables (yet).
Let me know if you want to see how to use them in a C++ program!
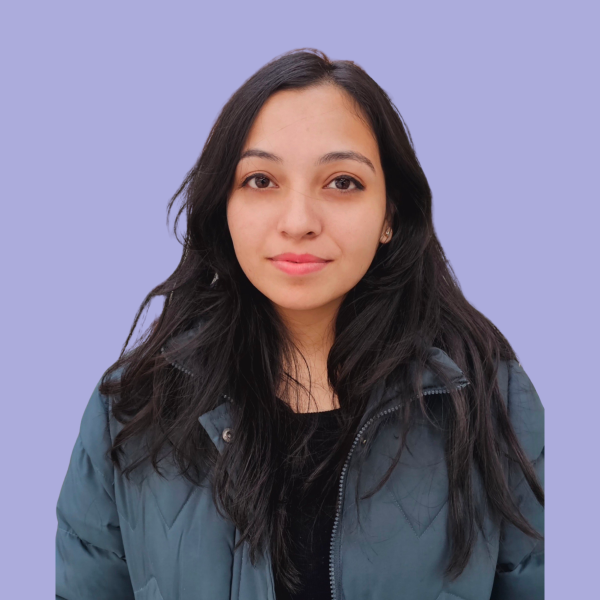
You can think of comments as helpful notes you leave in your code. They're not run by the compiler, they're just there to make the code easier to understand.
Right now, your code might be simple enough to follow without them, but as programs get more complex, comments become extremely useful, especially when someone else is reviewing your code.
Using comments early on is a great habit! They help you (and others) quickly understand what different parts of the code do, even after a long break.
Hope this helps! Feel free to ask more questions if you're curious.
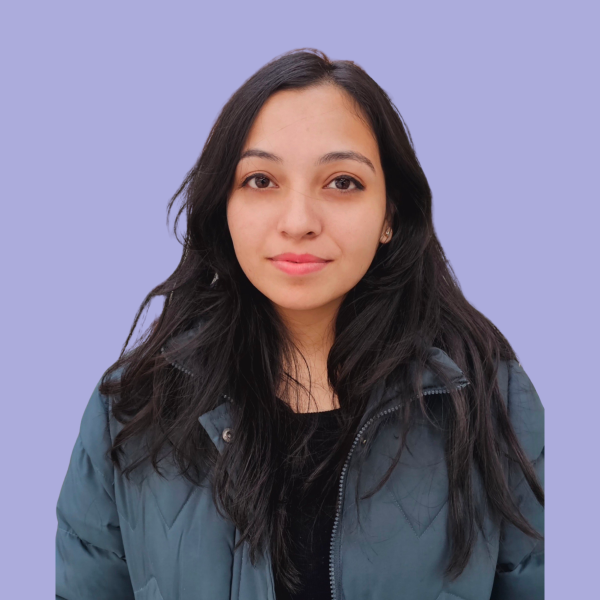
Good question!
Syntax in programming refers to the rules for how you write code, kind of like grammar in a language. If you don’t follow the rules (like missing a semicolon or a bracket), the computer won’t understand your code and will show an error.
It might feel a bit strict at first, but once you get the hang of it, it becomes second nature as you progress in your coding journey.
Let me know if you have more questions!
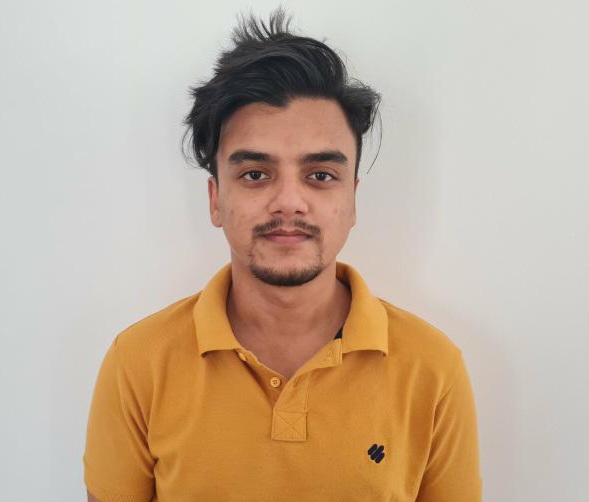
Hi Michael! That's a great question!
When converting kilometers to miles, we use the conversion factor:
1 kilometer = 0.621 miles
Since 0.621 is a decimal, there’s a high chance that both the input (kilometers) and the output (miles) could involve decimal values. That’s why it's better to use the double
data type for both variables.
In short, use double
when you feel the value can include a decimal part, on the other hand, use int
when you feel the value cannot include a decimal part (like an age).
I hope this clarifies things for you! Let me know if you have any more questions; I'm here to help.
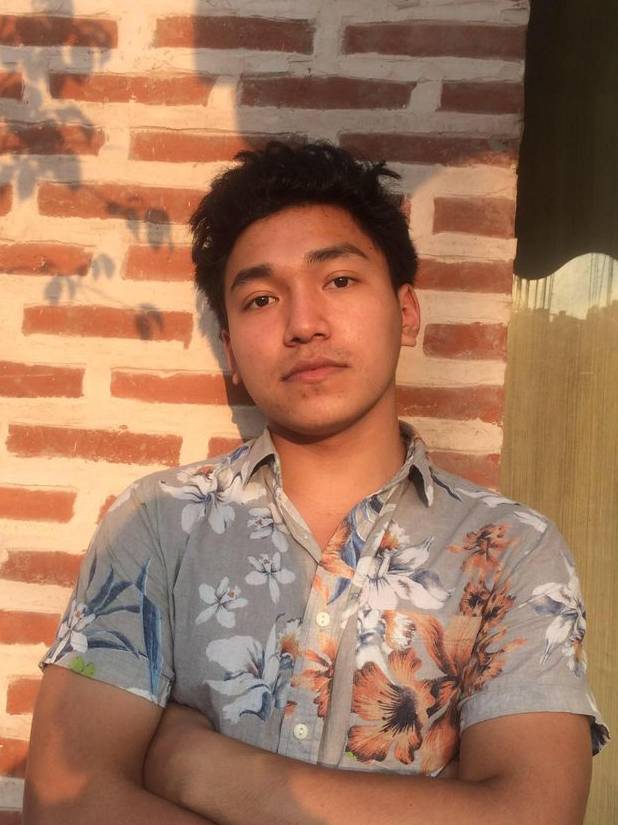
Scalable code is code that can handle growth, like more users, more data, or bigger problems, without needing to be completely rewritten.
Let me explain with a simple example:
Imagine you're building a house using LEGO blocks.
At first, the house is built for a small family of four, so just a few blocks are enough.
But what if the family grows?
If you’ve made the house scalable, you can add more rooms and floors just by snapping on more blocks.
However, if it wasn’t built with that flexibility, you'd have to tear the whole thing down and start over, which takes time and effort.
Similarly in coding:
If your code was originally designed to handle 100 users, and tomorrow that grows to 1,000 or even 100,000, you should be able to scale it up smoothly, without rewriting everything from scratch.
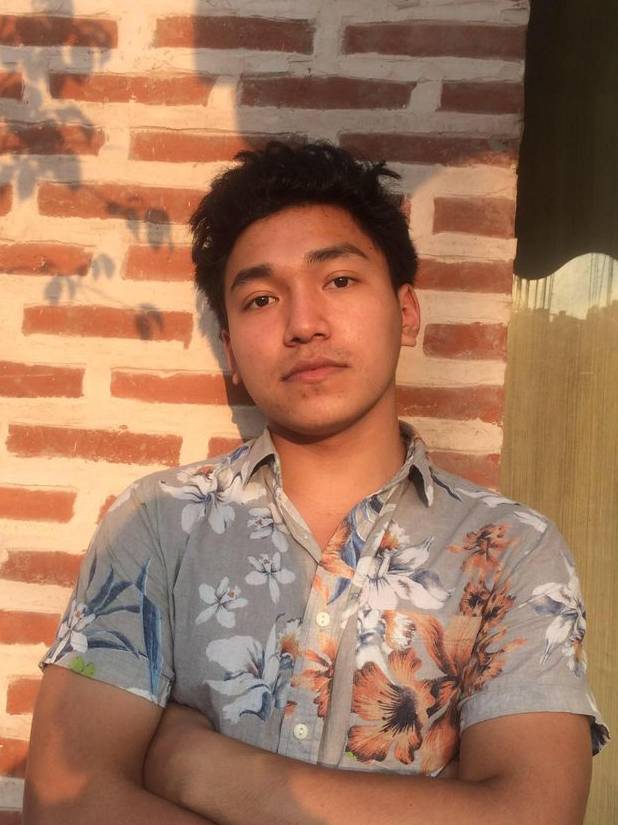
You're absolutely right that both else if
and
switch
can be used to handle multiple conditions, and it often
comes down to personal preference or the situation.
However, there are a few advantages to using switch
:
-
Cleaner code: When you're comparing a single variable to many possible values,
switch
often results in cleaner and more readable code than multipleelse if
statements. -
Efficiency: In some cases,
switch
can be more efficient. When you have many conditions to check for the same variable,switch
can sometimes make the process faster than evaluating each condition one by one aselse if
does.
Here’s a simple example in C++ to show the difference:
// Using else if
#include
using namespace std;
int main() {
int day = 3;
if (day == 1) {
cout << "Monday";
} else if (day == 2) {
cout << "Tuesday";
} else if (day == 3) {
cout << "Wednesday";
} else {
cout << "Another day";
}
return 0;
}
In this example, each condition is checked one by one until a match is found.
// Using switch
#include
using namespace std;
int main() {
int day = 3;
switch(day) {
case 1:
cout << "Monday";
break;
case 2:
cout << "Tuesday";
break;
case 3:
cout << "Wednesday";
break;
default:
cout << "Another day";
}
return 0;
}
Here, the switch
allows the program to directly jump to the
matching case
block, which can be more efficient and helps the
logic feel more organized.
Both approaches work well, and it’s good to be comfortable with both. When
dealing with a single variable and multiple constant values,
switch
tends to be a cleaner and often faster choice.
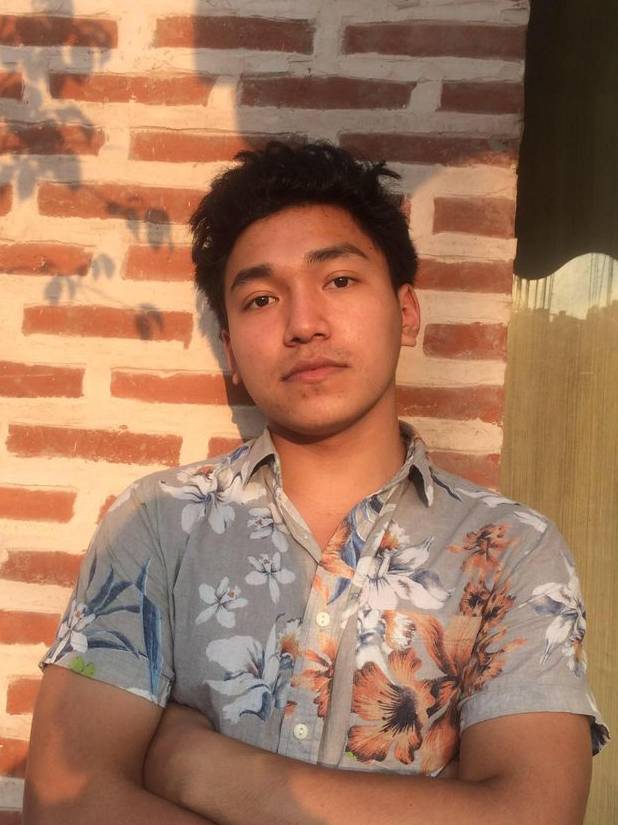
The main difference between arrays and vectors is that an array is a fixed-size collection of elements, whereas a vector can grow or shrink as needed.
Both are used to store multiple values, but they work a bit differently.
With arrays, once you define the size, it can't be changed. For example:
int arr[5];
Here, you've got space for exactly 5 integers—no more, no less. If you later want to store 6 or more values, you'll need to create a new array and manage copying manually.
Vectors, on the other hand, are part of the C++ Standard Library and are more flexible. You can start with an empty vector and keep adding elements using push_back()
:
#include
using namespace std;
vector nums; // Starts empty
nums.push_back(10); // Adds 10 to the vector
nums.push_back(20); // Adds 20
The vector automatically resizes itself in the background as you add elements—no need to specify the size in advance.
Note: If you're working with a known, fixed number of values and performance is critical, arrays can be slightly faster. But in most cases, vectors are preferred for their ease of use and flexibility.
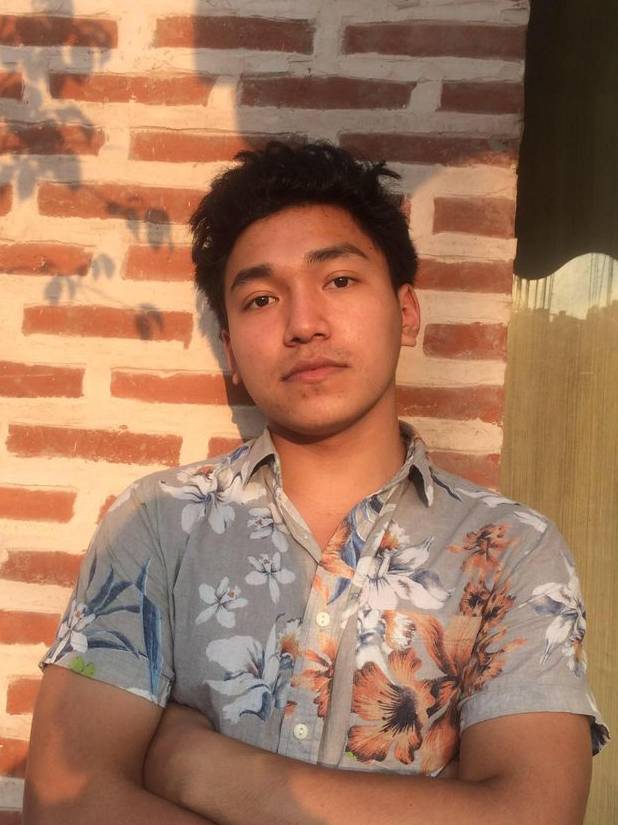
Yes, you can technically use void main()
instead of int main()
. However, it's not recommended.
According to the C++ standard, the correct and portable way to define the main function is:
int main() {
// your code here
return 0;
}
This version is universally accepted and ensures that your program can properly communicate with the operating system. The return 0;
statement signals that the program finished successfully.
If you use void main()
, you won't be able to use return 0;
because the function doesn’t return anything. That might not cause an error in some compilers, but it's considered non-standard and could lead to unpredictable behavior or reduced portability.
In short:
Use
int main()
for standard, portable, and reliable C++ code.void main()
might work in some environments, but it’s not guaranteed.
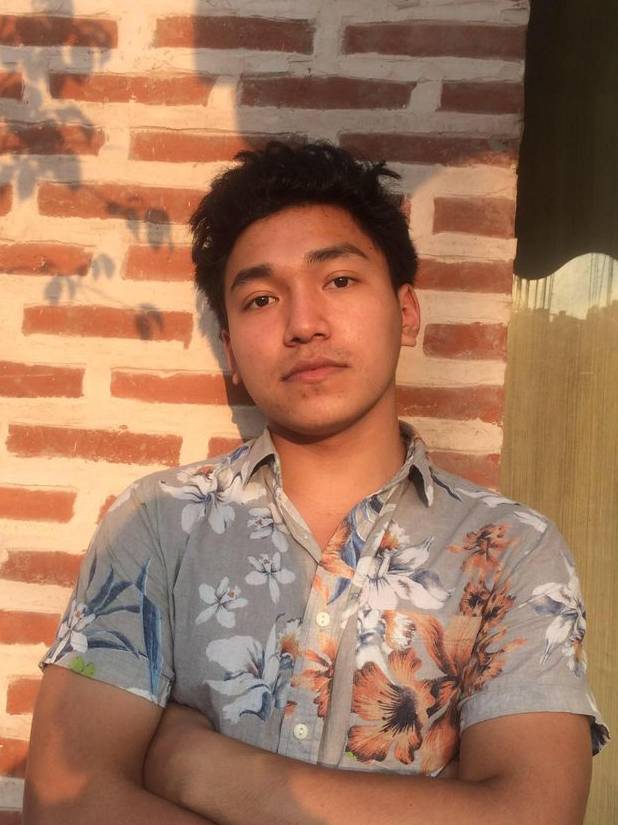
In the code:
#include
using namespace std;
int main() {
// create a variable
double number = 83.13;
// create a pointer variable
double* pt;
// assign address to pointer
pt = &number;
// print pointer pt
cout << pt;
return 0;
}
The reason the pointer needs to be a double*
is that the variable number
is of type double
.
In C++, the type of a pointer should always match the type of the variable it points to. So if number
were an int
, then the pointer should also be an int*
.
Here's a quick comparison to help make it clearer:
int a = 5;
int* ptr1 = &a; // OK: both are int
double b = 6.2;
double* ptr2 = &b; // OK: both are double
double* wrongPtr = &a; // Not OK: types don’t match (int vs double)
Using the correct pointer type ensures that the program knows how much memory to access and interpret properly. For instance, a double
typically uses more bytes than an int
, and a mismatch can lead to unexpected behavior.
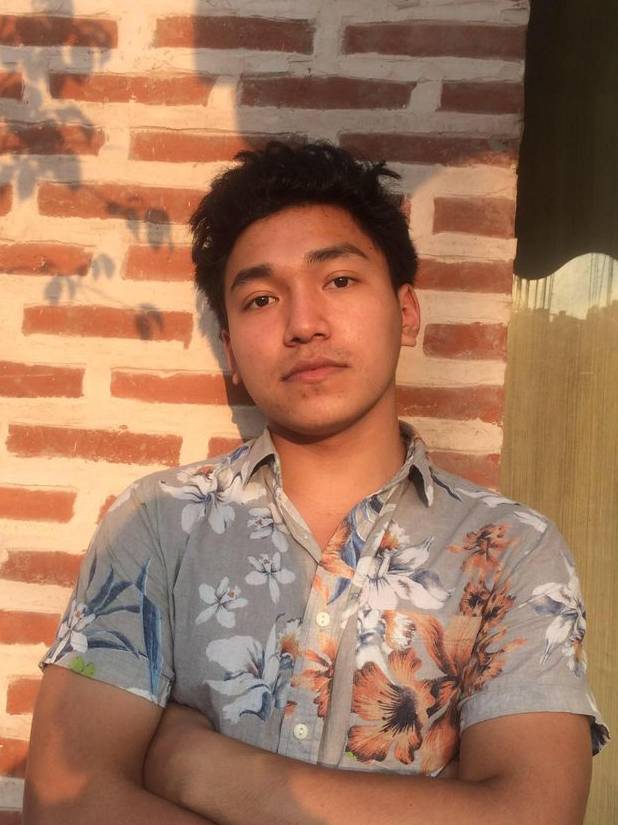
I agree that all the names listed in the good and bad variable name examples are valid options for C++ programs.
However, when defining good variable names, the goal is to choose names that are both easy to understand and easy to work with.
For example, it’s much clearer to understand a variable named salary
than one named s
. Similarly, age
is much easier to use and comprehend than something like aGekv2
, which doesn’t really convey any meaning.
In short, good variable names should be straightforward, intuitive, and consistent.