Ask Programiz - Human Support for Coding Beginners
Explore questions from fellow beginners answered by our human experts. Have some doubts about coding fundamentals? Enroll in our course and get personalized help right within your lessons.
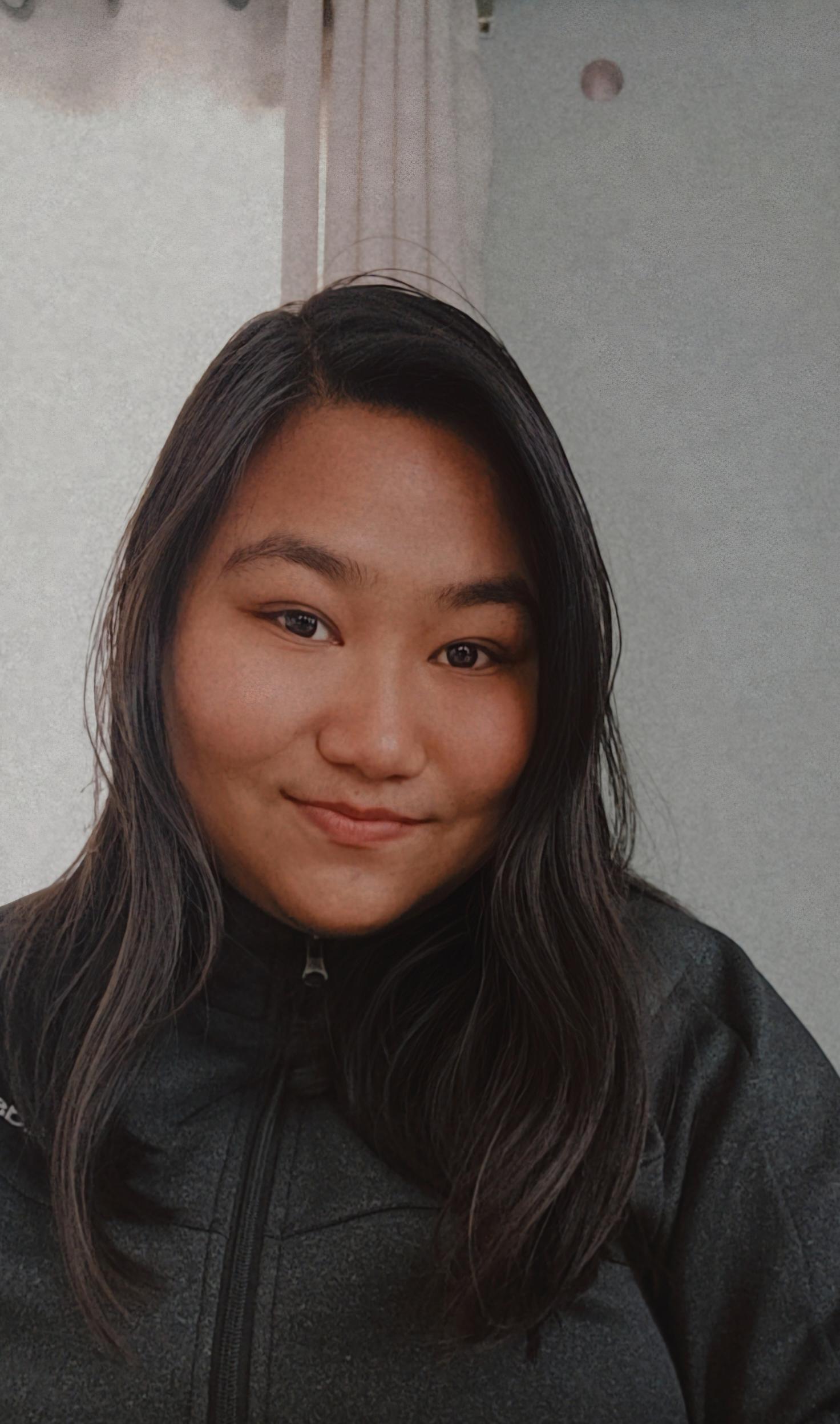
Yes, there’s an important difference between public class
and just class
in Java. Here’s what you need to know:
1. Accessibility Difference
public class
: The class can be accessed from anywhere in your program (even from other packages/folders).
public class Main { // Can be used everywhere
public static void main(String[] args) {
System.out.println("Hello!");
}
}
class
(no modifier): The class is only accessible within its own package/folder.
class Helper { // Only usable in this package
void doSomething() { ... }
}
2. File Naming Rule (For public Classes)
If you declare a class as public
, the filename must match the class name.
Name of the file containing
public class Main { ... }
should be Main.java.Name of the file containing
public class MyProgram { ... }
can't be Main.java.
3. When to Use Which?
Use
public
for classes that need to be shared across your project (likeMain
).Use default (
class
withoutpublic
) for helper classes that only one package uses.
// File: Main.java (Public, so filename matches)
public class Main {
public static void main(String[] args) {
Helper.help(); // Can only call if Helper is public or in same package
}
}
// File: Helper.java (No 'public' = package-private)
class Helper {
static void help() {
System.out.println("Assisting...");
}
}
Key Takeaway:
public
means anyone can use this class.No modifier means only my package/folder can use this class.
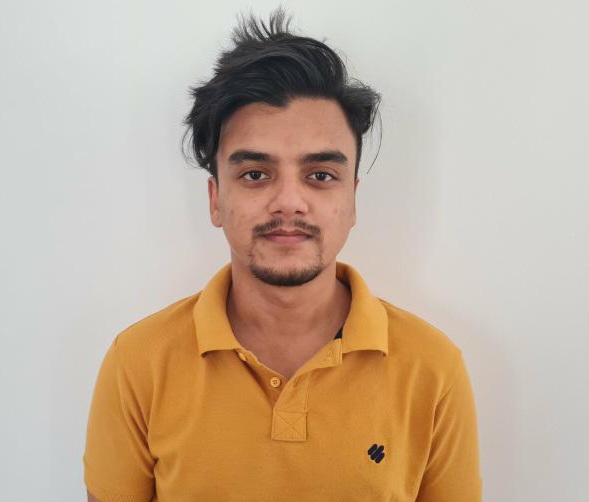
Hi there! for (int side : sides)
is a common way of using a for-each loop in Java to iterate over elements in an array or a collection.
Let's break it down:
sides
is an array of integers. In our context, it represents the lengths of the sides of a polygon.int side
declares that each element from thesides
array will be treated as an integer and namedside
during each iteration of the loop.for (int side : sides)
means "for each integerside
in thesides
array, do something..." In this case, we are summing all the side lengths to calculate the perimeter of a polygon.
This code snippet inside the loop:
perimeter = perimeter + side;
...adds the current side
value to the perimeter
. After iterating through all the sides in the array, the variable perimeter
will hold the total sum, representing the perimeter of the polygon.
Hope this helps clarify things! Feel free to ask if you have any more questions!
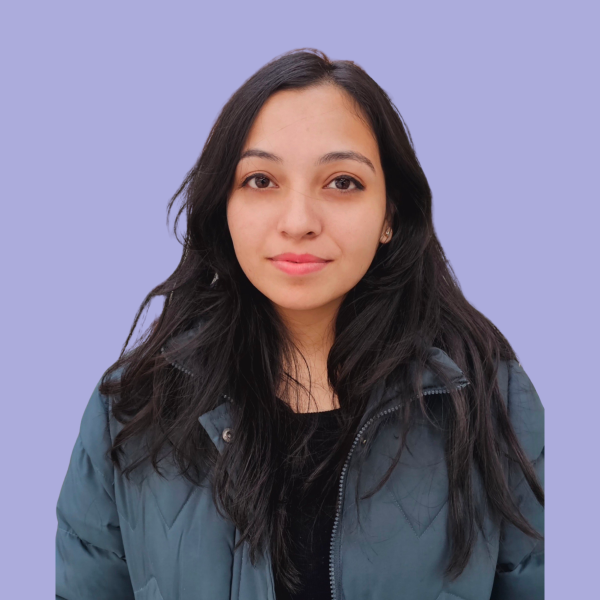
Good question!
When we say Java is machine-independent, it means that Java code can run on any computer, no matter what type it is. You don’t need to worry about whether it’s Windows, macOS, or Linux, Java works the same way on all of them.
This is possible because Java runs on a special program called the Java Virtual Machine (JVM) that takes care of everything for you. This platform independence is a major reason why Java is so widely used. It allows developers to write once and run anywhere (often referred to as "WORA").
Let me know if you have more questions about Java or anything else!
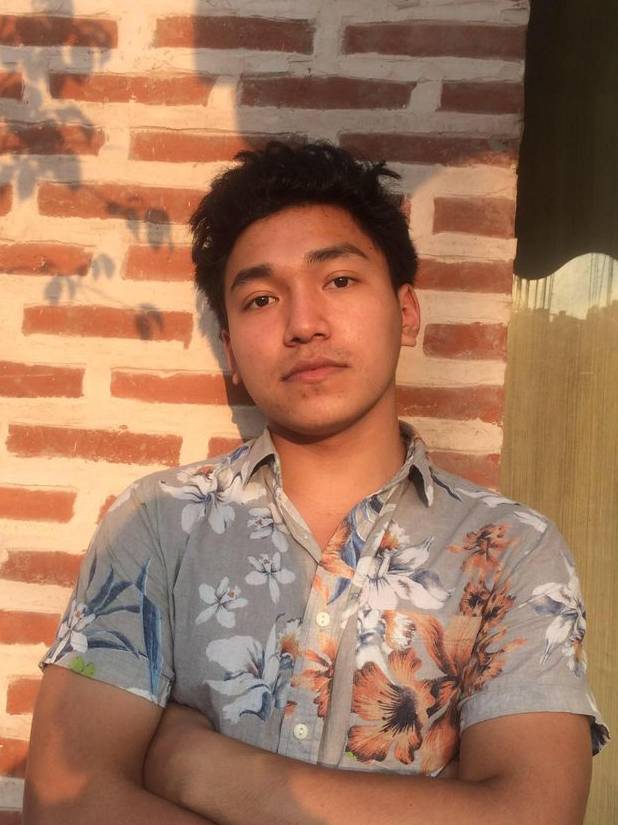
The break
statement is used to stop a loop based on certain conditions. It can be used to terminate any kind of loop—for
, while
, etc.
Here’s an example:
class Main {
public static void main(String[] args) {
for (int i = 1; i <= 10; i++) {
System.out.println("Hello Dhanush");
break;
}
}
}
Output
Hello Dhanush
According to the for
loop’s condition (i <= 10
), the loop is supposed to run 10
times. But because we’ve used break
right after the first print statement, the loop stops immediately after the first iteration.
Now let’s say we want to stop the loop after 5
iterations instead of immediately:
class Main {
public static void main(String[] args) {
for (int i = 1; i <= 10; i++) {
System.out.println("Hello Dhanush");
if (i == 5) {
break;
}
}
}
}
Output
Hello Dhanush
Hello Dhanush
Hello Dhanush
Hello Dhanush
Hello Dhanush
Here, Hello Dhanush
is printed 5
times. That’s because we’ve used the condition if (i == 5)
to break out of the loop when i
reaches 5
.
Note: break
is very useful when you want to exit a loop early, without having to wait for the loop's natural condition to end. You can apply this same logic in different scenarios, like stopping a search when the target is found.
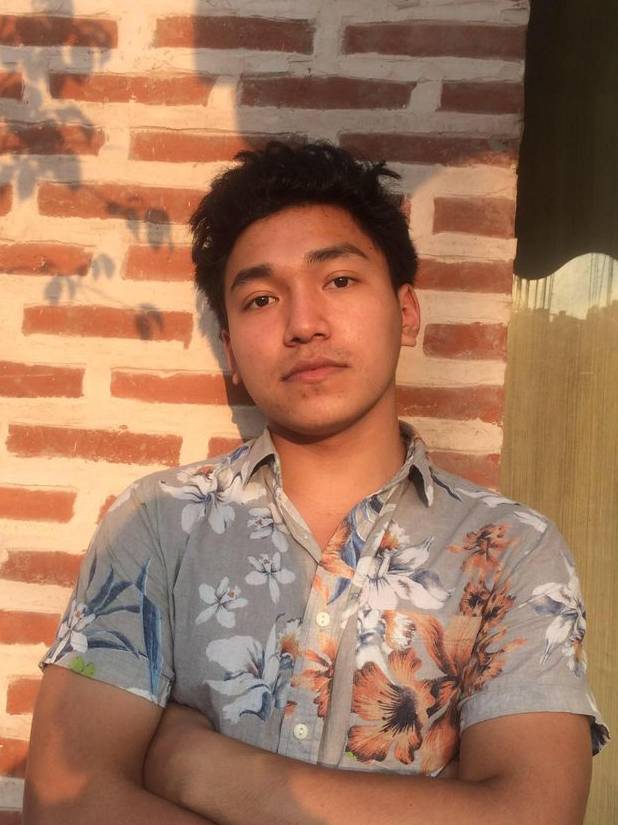
You're right that in JavaScript, there's not much difference between using single quotes (' '
) and double quotes (" "
), as both are used to define strings.
But in Java, there's an important distinction:
Single quotes are used for single characters — this data type is called
char
.Double quotes are used for strings, which can contain multiple characters.
Here's an example:
// Single character using single quotes
char letter = 'A';
// A string of characters using double quotes
String word = "Hello";
If you try to store multiple characters inside single quotes like this:
char letter = 'Hello'; // This will cause an error
Java will throw an error because char
can only hold a single character, not a sequence.
In short,
char
is meant for a single character (like'A'
,'b'
,'5'
, or'@'
).String
is meant for text that can have one or many characters (like"Hello"
,"123"
,"Good morning"
).
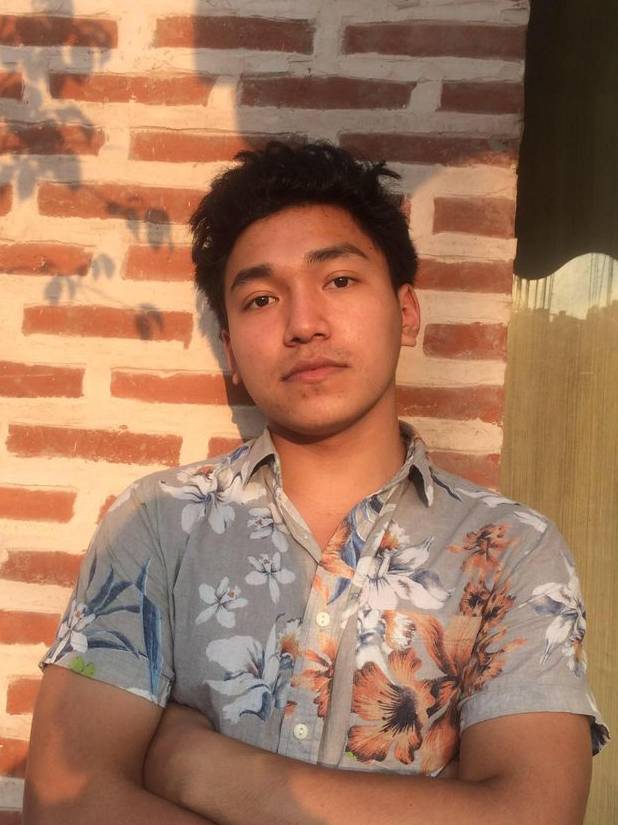
I understand that you're hearing mixed opinions about Java.
For beginners, Java can feel a bit overwhelming at first, mainly because it has more rules and structure compared to some other languages like Python. For example, in Python you can print something with just:
print("Hello")
But in Java, even the simplest program needs a full structure like this:
public class Main {
public static void main(String[] args) {
System.out.println("Hello");
}
}
This extra structure can feel like a lot when you're just starting out.
However, once you get the hang of it—just like with any other language—it becomes much easier and more natural to work with. You’ll soon feel comfortable using Java to write code.
Also, the structure and rules that might seem tough at first are actually what make Java so powerful and widely used in the industry. Its strictness helps avoid many bugs and makes large-scale software development more manageable.
Java is also used in a lot of real-world applications—from Android apps to banking systems—so the effort you put in can really pay off.
The key, as with anything, is to take it step by step. Once you're comfortable with the basics, it’ll start making more sense. It might feel challenging at the start, but with practice, it’ll definitely get smoother.
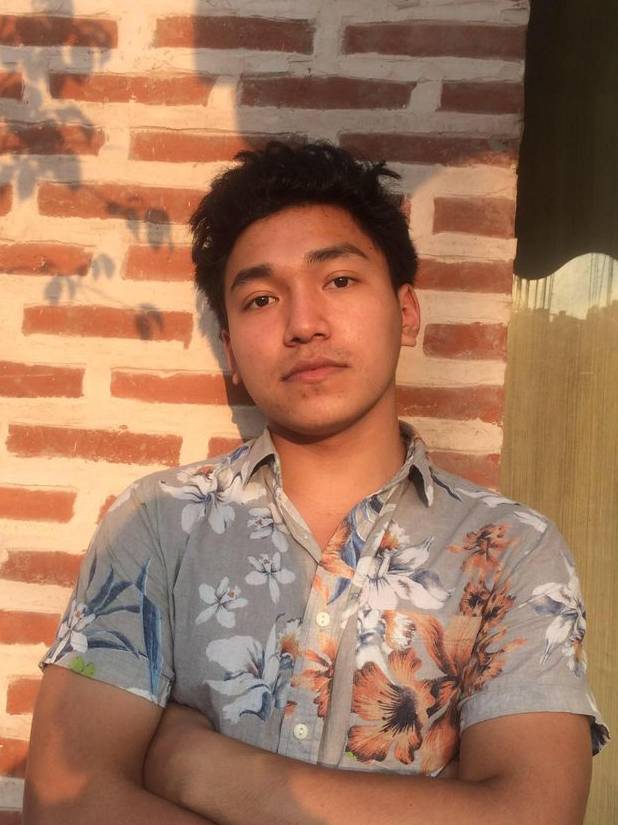
Coding is a lot like mathematics—the best way to memorize it is through consistent practice.
Try writing small pieces of code regularly and experiment with different examples. The more you practice, the more familiar the concepts will become, and the easier it'll be to remember them.
Also, try breaking things down into smaller chunks. Instead of trying to learn everything at once, focus on one concept at a time and practice it until it feels comfortable.
Don't worry if you forget something; it’s completely normal. With time, things will start to click.
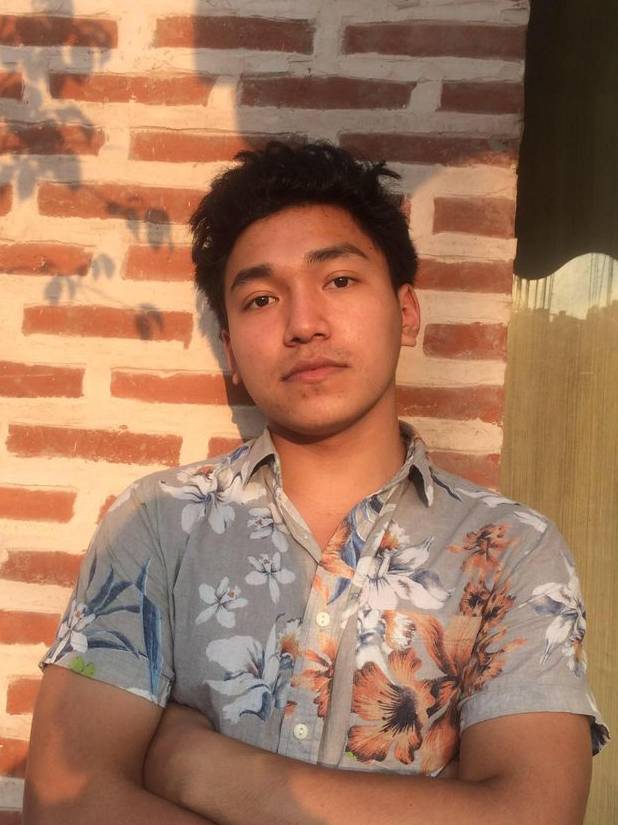
Yes, a double
in Java can store both whole numbers (integers) and decimal numbers (floating-point values).
For example:
// Store an integer
double num1 = 10;
// Store a decimal number
double num2 = 10.5;
Note that even though 10
is an integer, Java automatically converts it to 10.0
when stored in a double
variable.
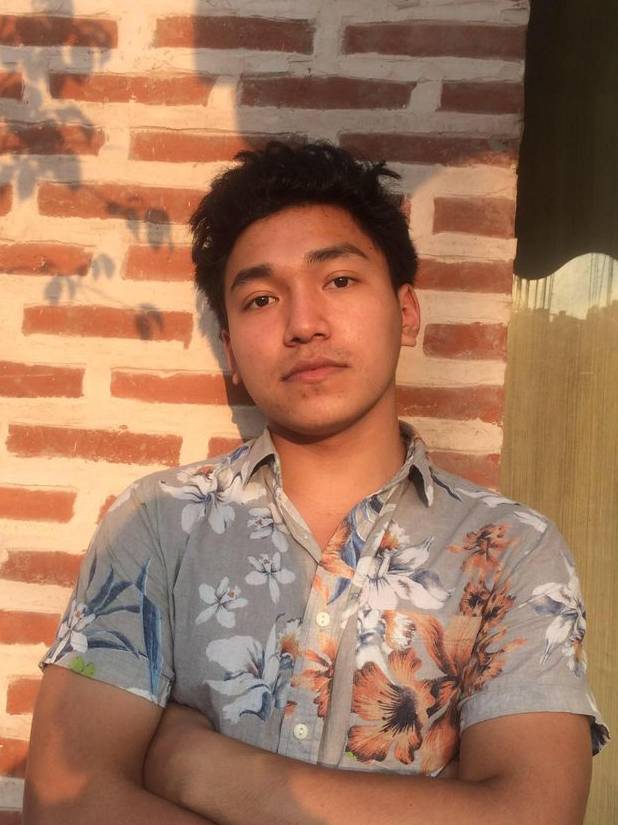
A HashMap
is not ordered in Java. It doesn’t guarantee any specific order of elements when you add or retrieve them. The reason for this is that HashMap
is optimized for fast lookups, and ordering elements doesn’t contribute to its primary goal.
If you need an ordered version, you have two options:
1. LinkedHashMap
: This data structure maintains the order of insertion. That means the order in which you add elements to the map will be preserved when you iterate over it.
Example:
Map linkedMap = new LinkedHashMap<>();
linkedMap.put("one", 1);
linkedMap.put("two", 2);
linkedMap.put("three", 3);
// Output will be in insertion order
for (Map.Entry entry : linkedMap.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
2. TreeMap
: This data structure automatically sorts the keys in natural order (or by a custom comparator if provided). The elements will be sorted based on the key.
Example:
Map treeMap = new TreeMap<>();
treeMap.put("banana", 2);
treeMap.put("apple", 1);
treeMap.put("cherry", 3);
// Output will be in sorted order by key
for (Map.Entry entry : treeMap.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
So, if you want ordered behavior, you’d use either LinkedHashMap
for insertion order or TreeMap
for sorted order.
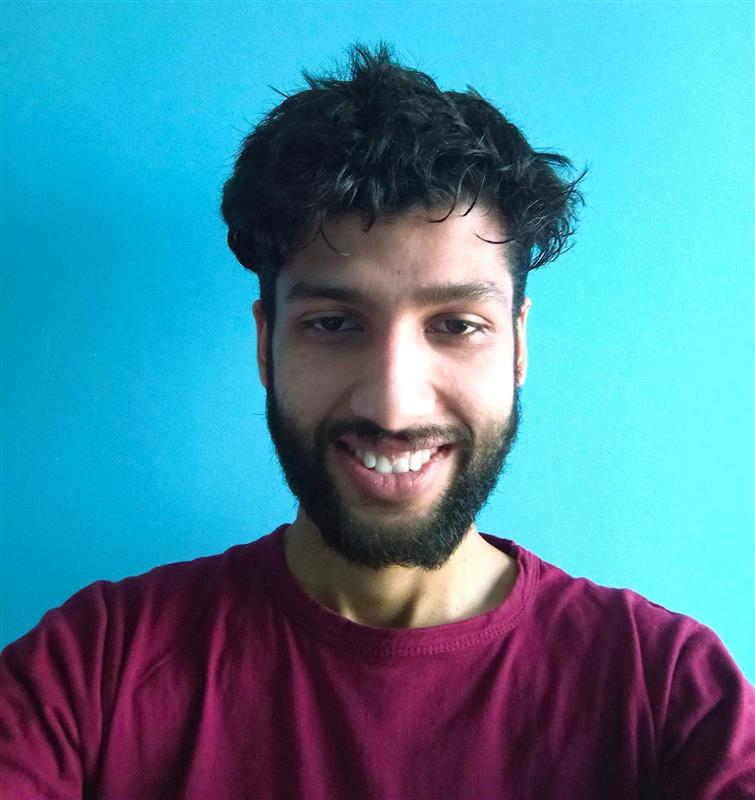
Java code can run on any operating system without needing changes because of a key component called the Java Virtual Machine (JVM).
Think of the JVM as a translator or a middle layer. Here's how it works:
When you write Java code, it’s compiled into an intermediate form called bytecode, not directly into machine code for a specific OS.
This bytecode is platform-independent—meaning it's the same no matter where it's run.
The JVM on each device reads the bytecode and translates it into machine code that the specific operating system and hardware can understand.
Since each OS (Windows, macOS, Linux, etc.) has its own version of the JVM, your Java program doesn't need to change. The JVM handles all the OS-specific translation for you.
Example:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Once compiled, this code becomes bytecode in a .class
file. That same file can be run on any system that has a JVM installed—no changes required.
This is what people mean when they say "Write once, run anywhere", which is one of Java’s biggest strengths.